Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial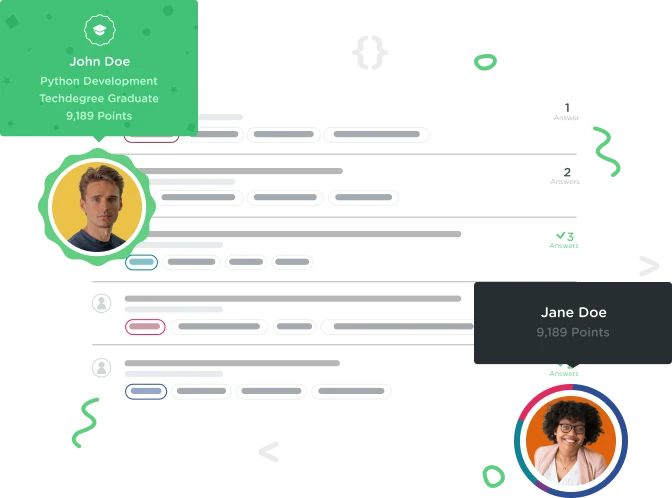
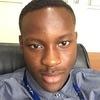
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsHow can I display my message?
SyntaxError: Unexpected token < in JSON at position 0.
My code:
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
// Require https module
const https = require('https');
const profileName = 'osaroigbinovia';
// Function which prints message to the console
function printMessage (profileName, badges, totalPoints) {
const message = `${profileName} has ${badges} badges and a total of ${totalPoints} points in the JavaScript programme.`;
console.log(message);
}
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${profileName}`, (response) => {
// Read the data
let body = '';
response.on('data', (info) => {
body += info.toString();
});
response.on('end', () => {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(profileName, profile.badges.length, profile.points.JavaScript);
});
});
4 Answers
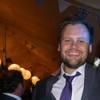
Tom Gooding
16,735 PointsHi Osaro,
You need to add .json to the url after the profileName variable. Otherwise you are requesting the HTML version of the page.
const request = https.get(`https://teamtreehouse.com/${profileName}.json`, (response) => {
// Read the data
let body = '';
response.on('data', (info) => {
body += info.toString();
});
response.on('end', () => {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(profileName, profile.badges.length, profile.points.JavaScript);
});
});
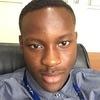
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsYou're using normal punctuation marks (' ') with interpolation e.g. ${username}, and it doesn't work like that in JavaScript. You need to use template literals i.e. backticks (``) when storing values in a variable you add in your statement(interpolating) e.g.
const message = `${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript`;
`https://teamtreehouse.com/${username}.json`
The backtick key is found just above the 'tab' key on a QWERTY keyboard and also put the 'use strict' statement at the top of all your code.
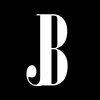
Joshua Bowden
Full Stack JavaScript Techdegree Graduate 29,312 PointsI am getting the same error but I have .json after my url:
// JavaScript Document
const https = require('https');
const username = "joshbowden2";
function printMessage(username, badgeCount, points) {
"use strict";
const message = '${username} has ${badgeCount} total badge(s) and ${points} points in JavaScript' ;
console.log(message);
}
printMessage("joshbowden2", 100 ,200);
//Connect to the API URL (https://teamtreehouse.com/joshbowden2.json)
const request = https.get('https://teamtreehouse.com/${username}.json', (response) => {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
// Parse the data
const profile = JSON.parse(body);
//console.dir(profile);
printMessage(username , profile.badges.length, profile.points.JavaScript);
// Print out info from API
});
});
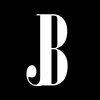
Joshua Bowden
Full Stack JavaScript Techdegree Graduate 29,312 PointsI knew those marks looked different. Thanks!
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsOsaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsThank you very much Tom, my code is working now.