Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial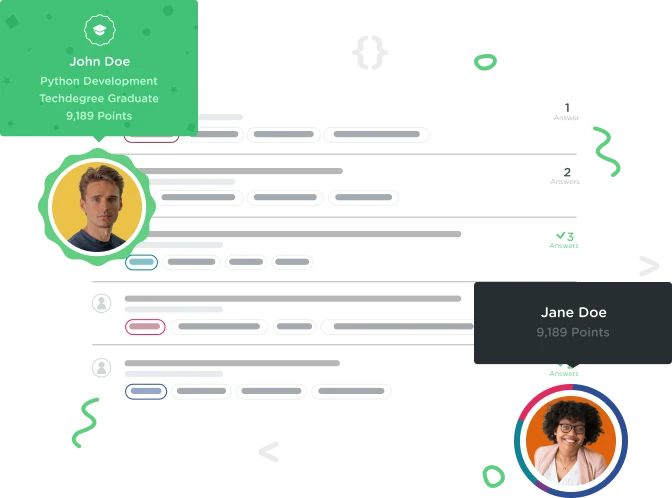

Radu - Adrian Buha
Courses Plus Student 5,535 PointsHow can I display the results of these queries in Visual Studio C#?
Hi,
I'm trying to do these queries in Visual Studio. How can I print/display the results of these queries in a Console Window?
For example, I have this code:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace Querying { class Program { static void Main(string[] args) { List<int> numbers = new List<int> { 2, 4, 8, 16, 32, 64};
var q = from number in numbers where number > 10 select number;
Console.WriteLine(q);
Console.ReadLine();
}
}
}
But when I run the app, I get these displayed: System.Linq.Enumerable+WhereListIterator'1[System.Int32] instead of {16, 32, 64}. Does anyone know a way?
Many thanks in advance!
4 Answers
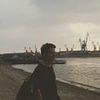
Calin Bogdan
14,921 PointsHi!
The query returns a list object. You have to process the elements in order to write them to the console that way.
for (int i=0; i < q.Length; i++)
{
Console.Write(q[i]);
}
Hope it helped!

Kiron Roy
3,210 PointsI cleaned up the code a little bit. Here is a link that is interactive: LINQ number list
Code below:
using System;
using System.Collections.Generic;
using System.Linq;
namespace LINQStuff
{
class Program
{
static void Main()
{
var numbers = new List<int> { 2, 4, 8, 16, 32, 64 };
var numberGreaterThanTen = from number in numbers where number > 10 select number;
foreach (int item in numberGreaterThanTen)
{
Console.WriteLine(item.ToString());
}
Console.ReadLine();
}
}
}
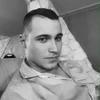
Dávid Polyovka
4,230 PointsDear Radu - Adrian Buha
var q = from number in numbers where number > 10 select number;
foreach (int item in numbers)
{
Console.WriteLine(item.ToString());
}
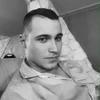
Dávid Polyovka
4,230 PointsYou have to use ToString() method. Hope it helped.

Radu - Adrian Buha
Courses Plus Student 5,535 PointsYeap! It worked. Thank you David!
Radu - Adrian Buha
Courses Plus Student 5,535 PointsRadu - Adrian Buha
Courses Plus Student 5,535 PointsServus Bogdan!
Still doesn't work. I get the same result... Any ideea why?
Edit: Found the issue, my mistake! I had the query sent ToString();
Thank you for your help!