Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial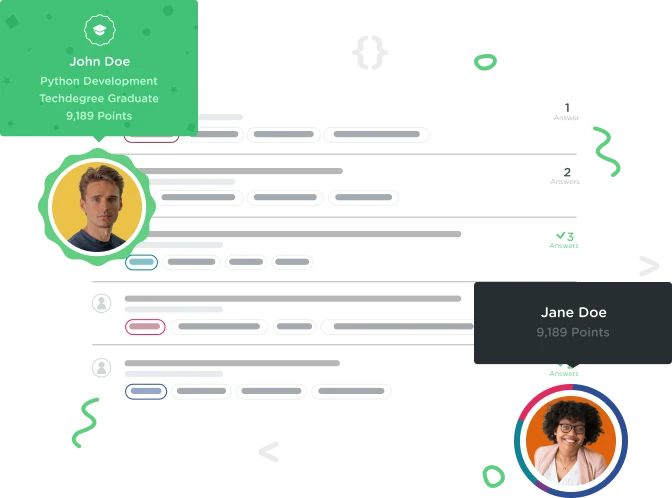
1 Answer
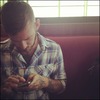
Erik McClintock
45,783 PointsYoussef,
Stipe is correct in the code that they have shared, but it might help you a bit more to walk through what is happening in the code so that you better understand how and why you construct it in this fashion.
Let's break it down piece by piece:
$names = array( 'Mike', 'Chris', 'Jane', 'Bob' );
In the above code snippet, we are declaring and instantiating an array, called $names
. A little bit of information about arrays...An array is a "data structure" that associates item values with keys, commonly referred to as "key => value pairs". In the array that we have above, we have forgone adding named keys to our items, and instead we allow their keys to default to their index numbers, which for arrays, start at the number zero and increment with each item. So, the value "Mike" exists at key index 0, the value "Chris" exists at key index 1, and so on. If we wanted to pull a particular value out of our array, say whichever value existed in the third slot (which would be the key index of 2, since we start counting at zero in arrays), we could say something like echo $names[2];
, which tells the computer to print whatever value exists at the 2nd index of the $names array to the screen.
Moving on:
foreach( $names as $name ) {
echo $name;
}
In the above code snippet, we are putting our $names
array to good use by iterating over each value that exists in it, and assigning the current value to a temporary variable called $name
so that we can do something with it, which in this case, is echoing that current value stored in the temporary variable $name
to the screen. It can help to read the loop in human-speech to understand it a little more easily: "for each item in $names, call them $name, and then echo that value." The loop will check the condition ($names as $name) once, then go inside the curly braces { } and follow whatever instructions exist there. It will then go back to the top of the loop to check the condition once again, and if there are still items in the $names array that we haven't looked at, we'll target that next item in the array, then go back inside the curly braces and follow the instructions, then rinse-and-repeat until we have targeted and echoed each value that exists in our $names array. The simple version of the structure for a foreach loop is:
foreach( $yourArrayNameHere as $whateverTempVariableNameYouWant ) {
// whatever you want to do for each item in your array here
}
Let's look at a more practical example...Let's say you have a list of favorite movies that you want to put on your website. At the moment, the list is only 5 movies long, but you know that the list will grow with time. With the number of movies being relatively small at the moment (at 5 items), you could just write them all out by hand and be done with it, as follows:
<ul class="movie-list">
<li class="movie-list__item">Back to the Future</li>
<li class="movie-list__item">The Dark Knight</li>
<li class="movie-list__item">The Empire Strikes Back</li>
<li class="movie-list__item">Toy Story</li>
<li class="movie-list__item">The Avengers</li>
</ul>
...but that's not very scalable, and it is very susceptible to human error. Imagine that you now have 100 favorite movies in your list...you can see how it could be tedious to repeat all that code by hand, and difficult to make sure you did it all correctly! Trying to hand-type each item over and over, or trying to copy/paste without introducing errors, is very difficult, especially as your number of items grows. Beyond that, imagine you have multiple developers working on the code. Maybe you're able to guarantee that you personally will never introduce an error by hand-writing each item or through copy/paste efforts, but can you guarantee that one of the other developers will be as dedicated to ensuring that quality? And, of course, "everyone makes mistakes."
Luckily, we have alternatives! We want our application to be dynamic and able to handle a varying amount of data at any given time, and to help protect our code from errors. Thus, to future proof our code, we'll create an array that will house our movie items, and then a foreach loop to print out the proper HTML structure, as well as the contents for each list item.
// create an array to hold the names of our favorite movies; we'll call the array "favoriteMovies"
$favoriteMovies = array( 'Back to the Future', 'The Dark Knight', 'The Empire Strikes Back', 'Toy Story', 'The Avengers' );
echo '<ul class="movie-list">'; // echo the opening ul HTML tag
foreach( $favoriteMovies as $movie ) { // create our foreach loop to go through every item in our array
echo '<li class="movie-list__item">' . $movie . '</li>'; // echo our li HTML tags and insert the value of our current $movie item in between them
}
echo '</ul>'; // make sure to close our ul HTML tag (outside of the foreach loop!)
Now, with this code in place, all anybody ever has to do is add new movies to the $favoriteMovies array (which can also be taken care of dynamically, through a function created elsewhere in your code, and/or through a user-facing form on your website, etc.), and voila! You have a much more flexible, secure code base to add your favorite movies to your website.
Hopefully this has helped to clarify some things for you! These are tricky concepts to understand, but with practice and repetition, you'll nail them down!
Happy coding!
Erik

Michael Oliver
7,855 Pointsvery nice explanation!! thanks for helping my brain
Stipe Stipic
17,520 PointsStipe Stipic
17,520 Pointshere is the code: <?php $names = array('Mike', 'Chris', 'Jane', 'Bob'); <br/>
foreach($names as $name){ <br/>
echo $name; <br/>
} <br>
?>