Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial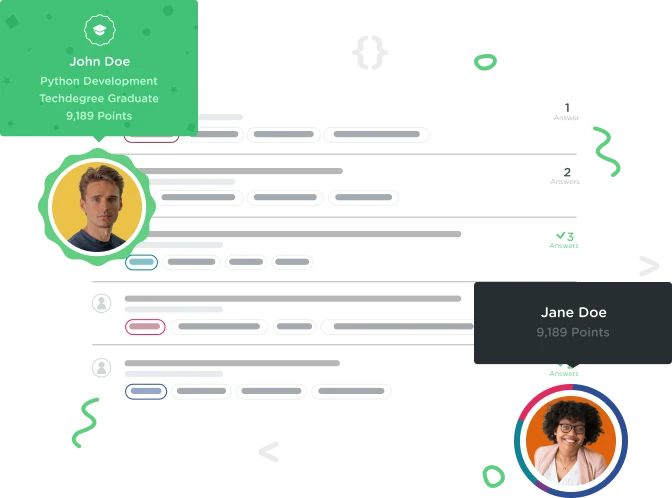
Miguel Nunez
3,266 PointsHow can I for loop this json example in AJAX?
How can I for loop a JSON object in AJAX. I asked this question on stack overflow but they are to strict and very sensitive people. I don't know how to add a code example on this site so here's the link https://stackoverflow.com/questions/45555534/how-can-i-for-loop-this-json-object-in-this-ajax-situation-not-a-duplicate?noredirect=1#comment78071136_45555534
2 Answers
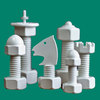
Steven Parker
231,269 PointsAt the link provided, there are two answers with two different loops that seem like they would do the job. Do they work for you?
And they both seem to be helpful. I didn't see any responses that appeared to be "strict and very sensitive ". I did notice that the question itself was downvoted a few times, but that might be a reaction to suggesting that many participants "suck at explaining" and asking for "no lazy people". I think the fact that folks answered anyway serves as a testament of the general good will in the community.
Miguel Nunez
3,266 PointsWell yeah I love the methods from both of the guys the one that I like the most and it's the easiest to understand and is closer to the tree house ways of doing thing was this guys code that I added to my file. But I notice his code had unlikable effects. I notice the button didn't disappear and every time I keep pressing the button it keeps generating the same JSON contents. In other words I want the JSON contents to show one time and I want the button to disappear after I press it so how can I do that?
Here's the AJAX file and JSON file ignore the three dots at the end of the JSON file.
<!--THE AJAX FILE-->
<!DOCTYPE html>
<html>
<body>
<div id="demo">
<button id="bg" type="button" onclick="x()">Change Content</button>
</div>
<script>
function x() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState == 4) {
var employees = JSON.parse(xhr.responseText);
for(var i=0;i<employees.length;i++){
employee = employees[i];
document.getElementById("demo").innerHTML += '<br>' + employee.name;
}
}
};
xhr.open("GET", "json_example_2.json", true);
xhr.send();
}
</script>
</body>
</html>
<!--THE JSON FILE-->
[
{
"name": "Adam",
"age": 21,
"interest": ["food", "relaxing"]
},
{
"name": "Bob",
"age": 22,
"interest": ["working out", "sleeping"]
},
{
"name": "Cane",
"age": 23,
"interest": ["football", "vide games"]
}
]
```
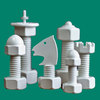
Steven Parker
231,269 PointsMiguel Nunez — I like the cute animation, how did you make that?
One easy way to make the button disappear is to set the display attribute to none. Expand the HTML to pass a reference to itself:
<button type="button" onclick="x(this)">Change Content</button>
And then in the script:
function x(btn) { // take the button argument
// ... do the other stuff ...
btn.style.display = "none"; // then make button vanish
}

GREGORY ASSASIE
3,898 PointsA quick fix for this is to remove all the textContent first each time, before the actually request is made.
<!--THE AJAX FILE-->
<!DOCTYPE html>
<html>
<body>
<div id="demo">
<button id="bg" type="button" onclick="x()">Change Content</button>
</div>
<script>
function x() {
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState == 4) {
var employees = JSON.parse(xhr.responseText);
document.getElementById("demo").innerHTML = "";
for(var i=0;i<employees.length;i++){
employee = employees[i];
document.getElementById("demo").innerHTML += '<br>' + employee.name;
}
}
};
xhr.open("GET", "json_example_2.json", true);
xhr.send();
}
</script>
</body>
</html>
<!--THE JSON FILE-->
[
{
"name": "Adam",
"age": 21,
"interest": ["food", "relaxing"]
},
{
"name": "Bob",
"age": 22,
"interest": ["working out", "sleeping"]
},
{
"name": "Cane",
"age": 23,
"interest": ["football", "vide games"]
}
]
```
GREGORY ASSASIE
3,898 PointsGREGORY ASSASIE
3,898 PointsThere is a viable response on your stackOverflow post. you have to parse the json before you can loop over it.