Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial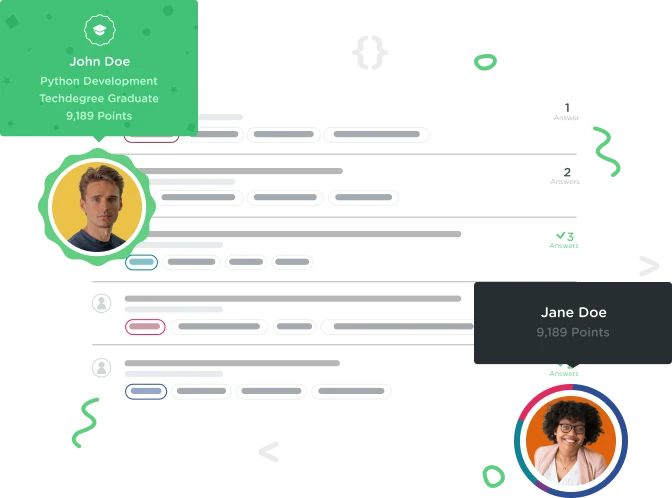

Daniel Kol Adam
3,285 PointsHow can I get the "Israel" answer to work whether the user types it in caps, none caps, or just one letter of caps?
This is my code:
var questions = [ ["What year is it?", "2018"], ["Where is Tel Aviv?", "Israel"], ["How many days in a week?", "7"] ];
var correctAnswers = 0; var question; var answer; var response; var html;
for (var i = 0; i < questions.length; i +=1) { question = questions[i][0]; answer = questions[i][1]; response = prompt(question);
if (response === answer) { correctAnswers += 1; } }
html = "You got " + correctAnswers + " questions correct"; document.write(html);
1 Answer
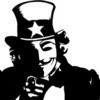
behar
10,799 PointsHey Daniel! You can use the .toLowerCase function. Say you have the following code:
var test = "QUIT"
test = test.toLowerCase()
test will now be "quit" instead of "QUIT", therefor just use the toLowerCase function on the prompt, so that everything they input is automaticly converted into lowercase, and then set the "Israel" to be lower case in the answer aswell. Hope this helps!
Daniel Kol Adam
3,285 PointsDaniel Kol Adam
3,285 PointsAnd how would you put it in this code? when I apply it to prompt, it applies it to all 3 answers in the loop, which suddenly gives me 0 correct answers.
behar
10,799 Pointsbehar
10,799 PointsKinda hard to tell you without supplying the code :P. However you cold simply do it like this:
var userInput = prompt("Input an answer").toLowerCase()
This code will convert whatever was inputted to all lowercase. And if you input non string it will do nothing. If you now set the "Israel" to just "israel" it will be the correct answer no matter how you inputted it eg:
So regardless of wether they input IsRaEl or Israel or ISRAEL, it will be converted to israel, and then tested!
Hope that helps, if you need more help though, please show me the updated code!
Daniel Kol Adam
3,285 PointsDaniel Kol Adam
3,285 PointsThis is the code
behar
10,799 Pointsbehar
10,799 PointsYes now simply add the .toLowerCase() function to the prompt and change "Israel" to "israel". Code:
Daniel Kol Adam
3,285 PointsDaniel Kol Adam
3,285 PointsThank you! I get it now !
behar
10,799 Pointsbehar
10,799 PointsGlad to help!