Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial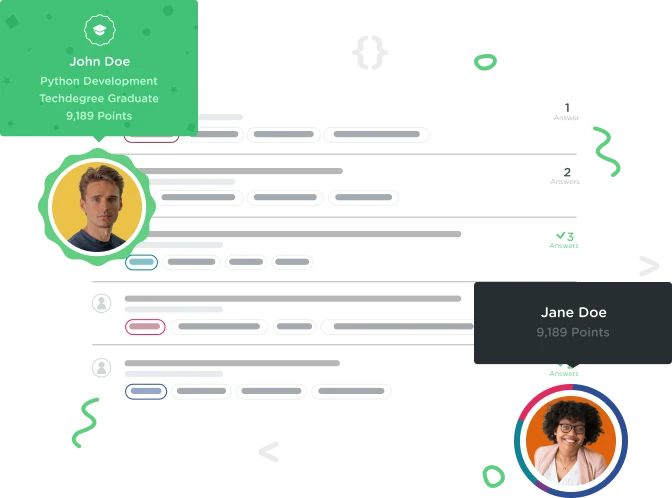
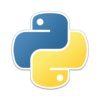
Luke Chulack
605 Pointshow can i get the monster to show up
after under standing how it works i think i got it done but i would like to add the monster to show up on the map or grid as M how can i do this?
2 Answers

Keerthi Suria Kumar Arumugam
4,585 PointsWhen you draw your map, you can check if the current cell that you are drawing matches with the monster's location and mark it with a "M" instead of a "|_|". I did something similar, where I showed the user, monster and the gate(dumb). The map also shows the sequence of steps that I have taken and displays it. Feel free to run the code.
import random
CELLS = [(0, 0), (0, 1), (0, 2), (0, 3),
(1, 0), (1, 1), (1, 2), (1, 3),
(2, 0), (2, 1), (2, 2), (2, 3),
(3, 0), (3, 1), (3, 2), (3, 3)]
def get_locations():
player = random.choice(CELLS)
monster = random.choice(CELLS)
gate = random.choice(CELLS)
if player == monster or gate == monster or player == gate:
player, monster, gate = get_locations()
return player, monster, gate
def get_m_locations(player,monster,gate):
monster1 = random.choice(CELLS)
if player == monster1 or gate == monster1 or monster == monster1:
monster1 = get_m_locations(player,monster,gate)
return monster1
def draw_map(pos,p_pos,m_pos,g_pos):
print(" _ _ _ _")
for num, item in enumerate(CELLS):
if num not in [3,7,11,15]:
if item == p_pos:
print("|X",end="")
elif item == m_pos:
print("|M",end="")
elif item == g_pos:
print("|G",end="")
else:
if item in pos.values():
for idx,item1 in pos.items():
if item == item1:
print("|{}".format(idx),end="")
break
else:
print("|_",end="")
else:
if item == p_pos:
print("|X|")
elif item == m_pos:
print("|M|",)
elif item == g_pos:
print("|G|",)
else:
if item in pos.values():
for idx,item1 in pos.items():
if item == item1:
print("|{}|".format(idx))
break
else:
print("|_|")
def avail_moves(p_pos):
x1,x2 = p_pos
st = "Press "
if not x1 == 0:
st = st + "W "
if not x1 == 3:
st = st + "S "
if not x2 == 0:
st = st + "A "
if not x2 == 3:
st = st + "D "
return st
def update_pos(p_pos,p_key):
x1,x2 = p_pos
if p_key == "W" and not x1 == 0:
x1 -= 1
elif p_key == "S" and not x1 == 3:
x1 += 1
elif p_key == "A" and not x2 == 0:
x2 -= 1
elif p_key == "D" and not x2 == 3:
x2 += 1
else:
print("ERROR CHOICE : Enter again")
return x1,x2
print("Welcome to the dungeon")
print("Get to the door to win while avoiding the monster")
print("The controls are W/S/A/D for UP/DOWN/LEFT/RIGHT")
p_pos,m_pos,g_pos = get_locations()
num = 0
pos = {num:p_pos}
while True:
#check if game is over
if p_pos == m_pos:
print("Sorry.. You have been gobbled by the monster")
break
elif p_pos == g_pos:
print("Yay.. You win.. You found the gate in {} steps".format(num))
break
else:
draw_map(pos,p_pos,m_pos,g_pos)
num += 1
print("You are in cell {}".format(p_pos))
print(avail_moves(p_pos))
p_key = input("> ").upper()
p_pos = update_pos(p_pos,p_key)
pos.update({num:p_pos})
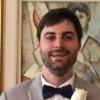
Chad Rappleyea
2,463 PointsCan someone explain how the code knows p_pos, m_pos, and g_pos correspond to the player, monster and gate respectively? I don't see the connection.