Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial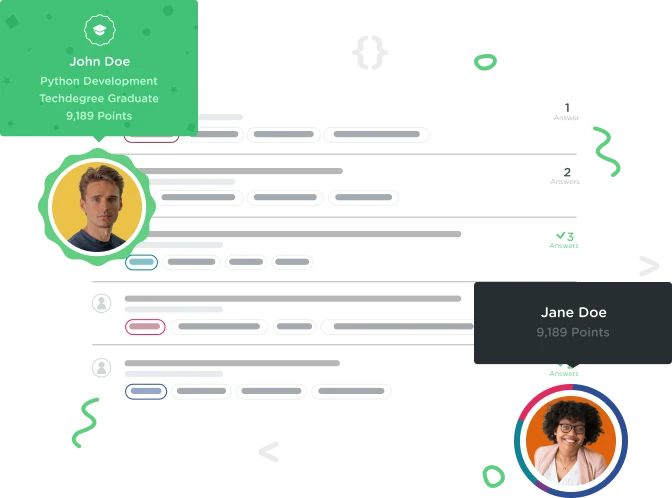
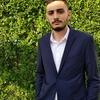
Vlad Tanasie
7,201 PointsHow can I get the value of a checkbox to know if it is checked or not and how can I change a prop of a MongoDB object?
I am trying to make my todo items state property change from active to completed when the checkbox is checked and then change the font to strikethrough. Should I make a separate function that uses the updated function or should I make a new route in express specifically for this?
When the todo is submitted it takes the 'active' state from the state property.
Also, how can I get the state of the checkbox, to know if it is checked or not?
<template lang="html">
<div>
<form v-on:submit='addTodo($event)'>
<input type='text' placeholder='Add or edit a todo' v-model='newTodo'/>
<textarea placeholder='Add or edit a description' v-model='newDescription'></textarea>
<input type='submit' />
</form>
<ul>
<li v-for='todo in todos' :key='todo._id'>
<input type='checkbox'>
<button @click="deleteTodo(todo._id)">X</button>
<p>{{todo.title}}</p>
<p>{{todo.description}}</p>
<button @click="updateTodo(todo._id)">Edit</button>
</li>
</ul>
</div>
</template>
<script>
import ToDoAPI from '@/services/ToDoAPI.js'
export default {
data () {
return {
newTodo: '',
newDescription: '',
state: 'active',
todos: []
}
},
mounted () {
this.loadTodos()
},
methods: {
async addTodo (evt) {
evt.preventDefault() // prevents the form's default action from redirecting the page
const response = await ToDoAPI.addTodo(this.newTodo, this.newDescription, this.state)
this.todos.push(response.data)
this.newTodo = '' // clear the input field
this.newDescription = '' //clear description
},
async loadTodos () {
const response = await ToDoAPI.getToDos()
this.todos = response.data
},
deleteTodo(todoID){
ToDoAPI.deleteTodo(todoID)
//remove the array element with matching id
this.todos = this.todos.filter(function(obj){
return obj._id !== todoID
})
},
//add an update function to update each todo
async updateTodo(todoID){
await ToDoAPI.updateTodo(todoID, this.newTodo, this.newDescription)
this.loadTodos()
this.newTodo = '' // clear the input field
this.newDescription = '' //clear description
}
}
}
</script>
<style lang="css">
textarea {
resize: none;
}
li{
border: 1px;
border-style: solid;
border-color: black;
margin-top: 10px;
}
</style>
//express setup
//the routes
//get the todos to display (READ)
app.get("/todo", (req, res) => {
const collection = client.db('todoapp').collection("todos"); //connecting to the atlas collection
collection.find().toArray(function (err, results){ //filtering the results
if(err){
console.log(err)
res.send([])
return
}
res.send(results)
})
})
//add a todo (CREATE)
app.post('/addTodo', (req, res) => {
const collection = client.db('todoapp').collection('todos')
let todo = req.body.todo //parse the data from the request body
let description = req.body.description
let state = req.body.state
collection.insert({title: todo , description: description , state: state}, function(err, results) {
if (err){
console.log(err);
res.send('');
return
}
res.send(results.ops[0]) //retruns the new document
})
})
//delete a todo (DELETE)
app.post('/deleteTodo', (req, res) => {
const collection = client.db('todoapp').collection('todos');
// remove the document by the unique id
collection.removeOne({'_id': mongo.ObjectID(req.body.todoID)}, function(err, results){
if(err) {
console.log(err)
res.send('')
return
}
res.send()
})
})
// add an update functio to update the existing mongodb data by its unique id
//update a todo (UPDATE)
app.post('/updateTodo', (req, res) => {
const collection = client.db('todoapp').collection('todos');
let todo = req.body.todo;
let description = req.body.description;
let state = req.body.state;
const updateOperation = {};
if(todo) updateOperation.title = req.body.todo;
if(description) updateOperation.description = req.body.description;
if(state) updateOperation.state = req.body.state;
collection.update({'_id': mongo.ObjectID(req.body.todoID)}, {$set: {...updateOperation}}, function(err, results){
if(err) {
console.log(err)
res.send('')
return
}
res.send()
})
})
//axios setup
import API from '@/services/API'
export default {
getToDos () {
return API().get('todo') // connecting to the api
},
addTodo (todo, description, state) {
return API().post('addTodo', {
todo: todo, // add our data to the response body
description: description,
state: state
})
},
deleteTodo (todoID) {
return API().post('deleteTodo', {
todoID: todoID // add data to the request body
})
},
updateTodo (todoID, todo, description) {
return API().post('updateTodo', {
todoID: todoID,
todo: todo,
description: description
})
}
}