Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial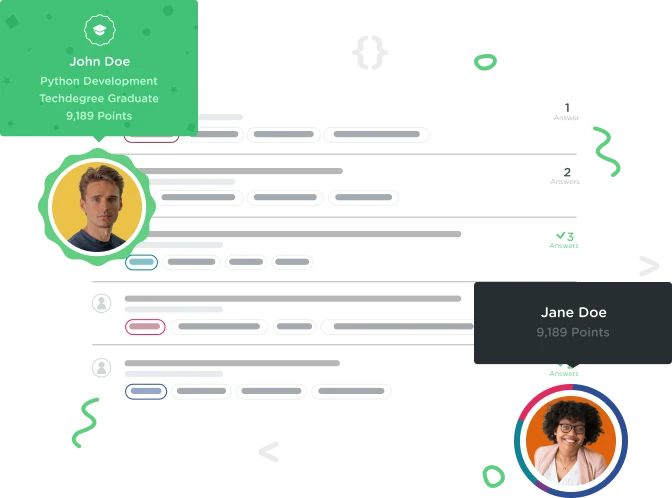

Andrea Tan
380 PointsHow can I have different responses for different Value Errors?
When running the masterticket.py code from the video with the ValueError as err, and I input a large number of ticket requests (e.g., 1000), then the value error output is valid. The console reflects the following:
"Oh no! We ran into an issue. Not enough tickets! Please try again."
But if I input a string value (e.g., "three"), which is what the ValueError was supposed to correct for originally, the output isn't as clean. It states the following:
"Oh no! We ran into an issue. invalid literal for int() with base 10: 'three' Please try again."
How can I code the program so that if the user inputs a string value, the error message is just "Oh no! We ran into an issue. Please try again." Or even better, incorporate an error message that indicates an integer needs to be used.
Here's my code:
while tickets_remaining >=1:
print("There are {} tickets remaining.".format(tickets_remaining))
username = input("What is your name?")
ticket_quantity = input("Hi {}, how many tickets would you like?".format(username))
try:
ticket_quantity = int(ticket_quantity)
if ticket_quantity > tickets_remaining:
raise ValueError("Not enough tickets!")
except ValueError as err:
print ("Oh no! We ran into an issue. {} Please try again.".format(err))
1 Answer
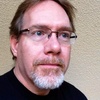
Chris Freeman
Treehouse Moderator 68,423 PointsThe issue is you are using the same except
statement to handle both errors.
The int
error raised will have its own message. It is best to narrow the try/except to the specific statement of concern. One for the int()
and another for the quantity. Though the quantity doesn’t really need a raise
statement. Rather just Print out the error message (and maybe reset quantity to a “fail value” like -1)
Then the while
could be
tickets_remaining >= 1 and ticket_quantity < 0
Post back if you need more help. Good luck!!!