Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial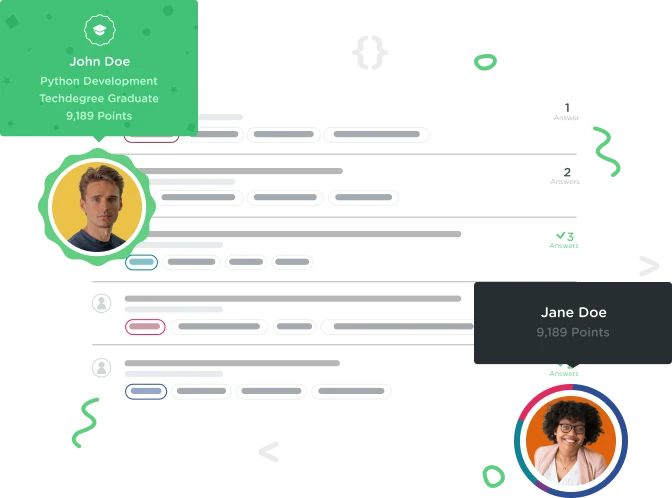
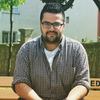
Arash Emadi
10,002 PointsHow can I have two separate SESSIONS in a CodeIgniter Web Application?
Hey Everyone,
I've been working on a web application for some time now. I use CodeIgniter to develop my web app. I've been facing a problem lately which I can't solve on my own.
I have designed an Authentication model which is used to authenticate my users when they log in. I have two login systems. One for the front-end and one for the back-end. My administrators log into the back-end and my site members log into the front-end to use the member-only features.
The problem is, whenever someone logs into the front-end, They get signed into the back-end as well (On the same computer), and if, for example, I want to only sign out of my account on the front-end, I also get kicked out of the back-end too! What I figure the problem is, is that CodeIgniter is using the same SESSION for both of these login systems.
Can someone please tell me how to have multiple sessions without them interfering? I don't want both my login sessions destroyed when one of the destroy_sess functions is called. I'd be grateful if you can help me. Below is parts of my PHP code.
MY MEMBERS CONTROLLER (FOR FRONT-END LOGIN):
<?php
/**
* Authentication Class
*/
class Members extends MY_Controller
{
public function login(){
// Validation Rules
$this->form_validation->set_rules('username','Username','trim|required|min_length[4]|xss_clean');
$this->form_validation->set_rules('password','Password','trim|required|min_length[4]|xss_clean');
if ($this->form_validation->run() == FALSE) {
// View
$this->load->view('layouts/login');
}else{
// Get From Post
$username = $this->input->post('username');
$password = $this->input->post('password');
// Validate Username & Password
$member_id = $this->Authenticate_model->login($username, $password);
if ($member_id != false) {
$user_data = array(
'user_id' => $member_id,
'username' => $username,
'logged_in' => true
);
// Set session user data
$this->session->set_userdata($user_data);
// Set Message
$this->session->set_flashdata('pass_login','You are now Logged In.');
redirect('login');
}else{
// Set Message
$this->session->set_flashdata('invalid_login','ERROR: Invalid Username or Password. Try Again.');
// View
$this->load->view('layouts/login');
}
}
}
public function logout(){
// Unset User Data
$this->session->unset_userdata('logged_in');
$this->session->unset_userdata('user_id');
$this->session->unset_userdata('username');
$this->session->sess_destroy();
// Set Message
$this->session->set_flashdata('logged_out','You have been Logged Out');
redirect('login');
}
}
?>
MY AUTHENTICATION CONTROLLER (FOR BACK-END LOGIN):
<?php
/**
* Authentication Class
*/
class Authenticate extends MY_Controller
{
public function login(){
// Validation Rules
$this->form_validation->set_rules('username','Username','trim|required|min_length[4]|xss_clean');
$this->form_validation->set_rules('password','Password','trim|required|min_length[4]|xss_clean');
if ($this->form_validation->run() == FALSE) {
// View
$this->load->view('admin/layouts/login');
}else{
// Get From Post
$username = $this->input->post('username');
$password = $this->input->post('password');
// Validate Username & Password
$user_id = $this->Authenticate_model->login($username, $password);
if ($user_id != false) {
$user_data = array(
'user_id' => $user_id,
'username' => $username,
'logged_in' => true
);
// Set session user data
$this->session->set_userdata($user_data);
// Set Message
$this->session->set_flashdata('pass_login','You are now Logged In. Welcome to the Administration panel.');
redirect('admin/dashboard');
}else{
// Set Message
$this->session->set_flashdata('invalid_login','ERROR: Invalid Username or Password. Try Again.');
// View
$this->load->view('admin/layouts/login');
}
}
}
public function logout(){
// Unset User Data
$this->session->unset_userdata('logged_in');
$this->session->unset_userdata('user_id');
$this->session->unset_userdata('username');
$this->session->sess_destroy();
// Set Message
$this->session->set_flashdata('logged_out','You have been Logged Out');
redirect('admin/login');
}
}?>
MY AUTHENTICATE MODEL
<?php
/**
* Model
*/
class Authenticate_model extends CI_Model
{
public function login($username, $password){
//Secure Password
$enc_password = md5($password);
// Validate
$this->db->where('username',$username);
$this->db->where('password',$enc_password);
$result = $this->db->get('users');
if ($result->num_rows() == 1) {
return $result->row();
}else{
return false;
}
}
}
2 Answers

miguelcastro2
Courses Plus Student 6,573 PointsYou need to check to see if someone is logged in to either the front or back-ends before completely destroying the session:
<?php
// On the front-end, check to see if a user is also logged into the back-end admin
// Logout front end
$this->session->unset_userdata('logged_in_front');
$this->session->unset_userdata('user_id_front');
$this->session->unset_userdata('username_front');
// Check if user is logged into backend, if so do not delete session
if (!$this->session->userdata('logged_in')) {
$this->session->sess_destroy();
}
You would have to perform this check on the backend as well to make sure that if you are logged into the backend to not log you out of the front end.

miguelcastro2
Courses Plus Student 6,573 PointsEasiest fix for this is to put your admin site in its own sub-domain such as:
admin.mywebsite.com
Then have you front-end on:
This way each site has its own cookie and will not share the same session.
Alternate fix would be to have a different value for "logged_in" on the back-end then one the front end. Back-end could be "admin_logged_in" and front-end could just be "logged_in". Also, check for an "admin_logged_in" before running the $this->session->sess_destroy(); command.
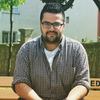
Arash Emadi
10,002 PointsHey. Thanks for your help. So I made some changes in my code. Now when I log in with a user in front-end, I don't get logged into the backend too, which is great! But when I logout anywhere, I get logged out everywhere, which is still a problem. Can you help me with this last part of my problem too?
- How can I destroy a specific SESSION?
- How can I have different SESSIONS and not just one, so when I destroy one, the others keep running?
<?php
/**
* Members Authentication Class
*/
class Members extends MY_Controller
{
public function login(){
// Validation Rules
$this->form_validation->set_rules('username','Username','trim|required|min_length[4]|xss_clean');
$this->form_validation->set_rules('password','Password','trim|required|min_length[4]|xss_clean');
if ($this->form_validation->run() == FALSE) {
// View
$this->load->view('layouts/login');
}else{
// Get From Post
$username = $this->input->post('username');
$password = $this->input->post('password');
// Validate Username & Password
$member_id = $this->Authenticate_model->login($username, $password);
if ($member_id != false) {
$user_data = array(
'user_id_front' => $member_id,
'username_front' => $username,
'logged_in_front' => true,
'name' => 'front'
);
// Set session user data
$this->session->set_userdata($user_data);
// Set Message
$this->session->set_flashdata('pass_login','You are now Logged In.');
redirect('login');
}else{
// Set Message
$this->session->set_flashdata('invalid_login','ERROR: Invalid Username or Password. Try Again.');
// View
$this->load->view('layouts/login');
}
}
}
public function logout(){
// Unset User Data
$this->session->unset_userdata('logged_in_front');
$this->session->unset_userdata('user_id_front');
$this->session->unset_userdata('username_front');
$this->session->sess_destroy();
// Set Message
$this->session->set_flashdata('logged_out','You have been Logged Out');
redirect('login');
}
}
<?php
/**
* Authentication Class
*/
class Authenticate extends MY_Controller
{
public function login(){
// Validation Rules
$this->form_validation->set_rules('username','Username','trim|required|min_length[4]|xss_clean');
$this->form_validation->set_rules('password','Password','trim|required|min_length[4]|xss_clean');
if ($this->form_validation->run() == FALSE) {
// View
$this->load->view('admin/layouts/login');
}else{
// Get From Post
$username = $this->input->post('username');
$password = $this->input->post('password');
// Validate Username & Password
$user_id = $this->Authenticate_model->login($username, $password);
if ($user_id != false) {
$user_data = array(
'user_id' => $user_id,
'username' => $username,
'logged_in' => true,
'name' => 'back'
);
// Set session user data
$this->session->set_userdata($user_data);
// Set Message
$this->session->set_flashdata('pass_login','You are now Logged In. Welcome to the Administration panel.');
redirect('admin/dashboard');
}else{
// Set Message
$this->session->set_flashdata('invalid_login','ERROR: Invalid Username or Password. Try Again.');
// View
$this->load->view('admin/layouts/login');
}
}
}
public function logout(){
// Unset User Data
$this->session->unset_userdata('logged_in');
$this->session->unset_userdata('user_id');
$this->session->unset_userdata('username');
$this->session->sess_destroy();
// Set Message
$this->session->set_flashdata('logged_out','You have been Logged Out');
redirect('admin/login');
}
}