Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial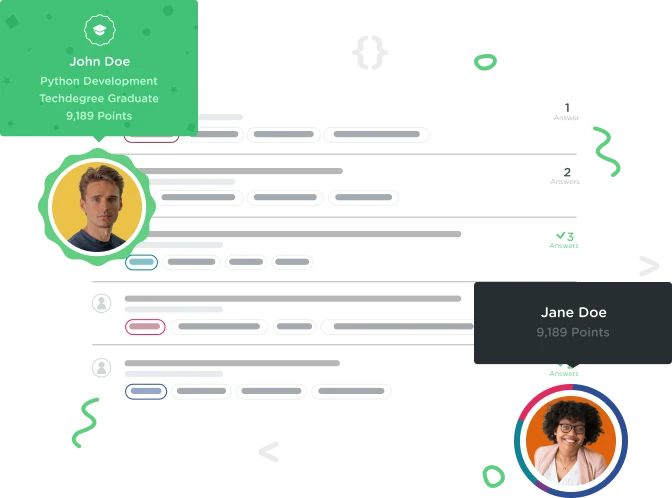
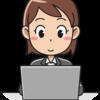
ilovecode
8,071 PointsHow can I improve my code?
I am not sure if I am allowed to ask this kind of question.
I am creating a basic online shop, where I have a .json file with the product details. I am using a fetch API to get the data from the json file and then display the products as a product listing with HTML generated. When I click on the 'Click for more details ' button then the product details display of the product. When I click on the 'Back button' I go back. It all works, but something is nagging on me that I can do better and that this possibly could be bad practice. If anyone can just give some suggestions on how to improve I will appreciate it so that I can go and try those methods.
const products = '../products.json';
let row = document.querySelector('.shop-body .row');
fetch(products)
.then(response => response.json())
.then(data => generateProduct(data));
function generateProduct(data) {
let prod = data.map(dat => {
return html = `<div id="product-${dat.id}" class="col-md-4 col-sm-12">
<div class="shop-list">
<a class="product-image">
<img src="../images/shops/${dat.image}" class="img-fluid" alt="${dat.product}">
</a>
<h4 class="product-name">${dat.product}</h4>
<button class="details">Click for more details</button>
</div>
</div>`;
});
row.innerHTML = prod.join('');
generateProductDetails(data);
}
function generateProductDetails(data) {
let detailBtn = document.querySelectorAll('.details');
let id = '';
for (let i = 0; i < detailBtn.length; i++) {
detailBtn[i].addEventListener('click', (e) => {
e.preventDefault();
id = data[i].id
if (data[i].id === id) {
row.innerHTML = `<div class="col-md-12 col-sm-12">
<div class="shop-list">
<button class="back">Back</button>
<h5 class="product-name">${data[i].product}</h4>
<p class="product-description">${data[i].description}</p>
<p class="product-price">${data[i].price} Galleons</p>
<p class="stock">In stock? ${data[i].stock}</p>
<p class="quantity">Quantity left: ${data[i].quantity}</p>
</div>
</div>`
}
goBackBtn(data);
});
}
}
function goBackBtn (data) {
let backBtn = document.querySelector('.back');
backBtn.addEventListener('click', (e) => {
generateProduct(data);
});
}
HTML
<div class="row"></div>
Sample JSON:
[
{
"shop": "Sweets",
"id": 1,
"product": "Sherbet",
"description": "Lick your fingers to get sweet and sour taste",
"stock": true,
"quantity": 12,
"image": "sherbet.jpg"
},
{
"shop": "Sweets",
"id": 2,
"product": "Toffee Apple",
"description": "Caramel with apple dipped in",
"stock": true,
"quantity": 5,
"image": "toffee-apple.jpg"
},
{
"shop": "Sweets",
"id": 3,
"product": "Chocolate",
"description": "Delicious and creamy",
"stock": true,
"quantity": 32,
"image": "chocolate.jpg"
},
{
"shop": "Sweets",
"id": 4,
"product": "Marshmallows",
"description": "Soft and fluffy",
"stock": true,
"quantity": 7,
"image": "marshmallows.jpg"
},
]
Thanks
1 Answer
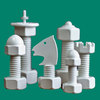
Steven Parker
231,210 PointsFor concerns about what is allowed, you can refer to the Terms of Service and the Code of Conduct. But I don't think either is at issue here.
The code itself seems pretty clean. The one issue that stands out is that the implicitly global "html" variable created in the "generateProduct" function isn't needed, and the callback can simply return the string directly. The compact form of the arrow function would work well here (leave off the braces and the "return").
Also, there are no "price" values in the products.