Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial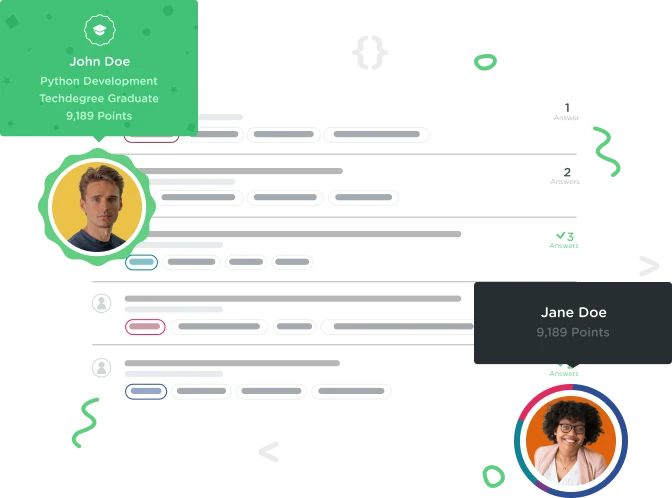

dylan kane
2,772 PointsHow can I make a field in a meteor Simple Schema equal to a js variable and more...
I am making a meteor web app where the user will click on a html button. Once this button is clicked, the user needs to be directed to another page with some forms generated by a meteor simple schema package. The first field in the simple schema needs to automatically be given a string value of "hello" and then the rest of the fields in the simple schema will be filled out by the user with the input fields on the page. What I am unsure about is how to get the first value automatically set to this string value. Here is some of the code I have:
The simple schema declaration:
LobbySchema = new SimpleSchema({
game: {
type: String,
label: "Game"
},
console: {
type: String,
label: "Console"
},
players: {
type: Number,
label: "Players"
},
mic: {
type: Boolean,
label: "Mic"
},
note: {
type: String,
label: "Note"
},
gamertag: {
type: String,
label: "Gamertag"
},
createdAt: {
type: Date,
label: "Created At",
autoValue: function(){
return new Date()
},
autoform: {
type: "hidden"
}
}
});
The first field there in the schema "game" needs to be given the value "hello" when the html button is clicked. Right now I can assign that value to a javascript variable using the button by having an onclick function:
function getElementText(elementID){
var elementText = "hello";
}
The button would call the getElementText function and have the elementText variable equal "hello". Now I need to assign the the first field in the simple schema to this variable value, "hello", then have it so the user can now fill out the rest of the schema with the input fields, automatically generated into the html with this code:
{{> quickForm collection="Lobby" id="insertLobbyForm" type="insert" class="newLobbyForm"}}
If you do not feel like providing the answer (maybe it happens to be more complicated than I think) then I would be very happy to receive a link to a site that might help me with this. I am also very willing to explain anything about the question if I did not explain the situation well enough above.
1 Answer

Joey Ward
Courses Plus Student 24,778 PointsIf I'm understanding correctly, you're looking at the first object "game" and looking to change the value of "label" from "Game" to "hello"?
You can modify this value by traversing through your LobbySchema object. So LobbySchema is an object which has several properties, one of which is called "game". The "game" property is also an object with two properties, one of which is "label".
To modify this value, you could use dot notation to access the variable and set a new value.
function htmlButtonClicked(){
LobbySchema.game.label = "hello";
}
dylan kane
2,772 Pointsdylan kane
2,772 PointsNo the label game is just to give the input field context when it is generated. Are you familiar with meteor simple schema?