Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial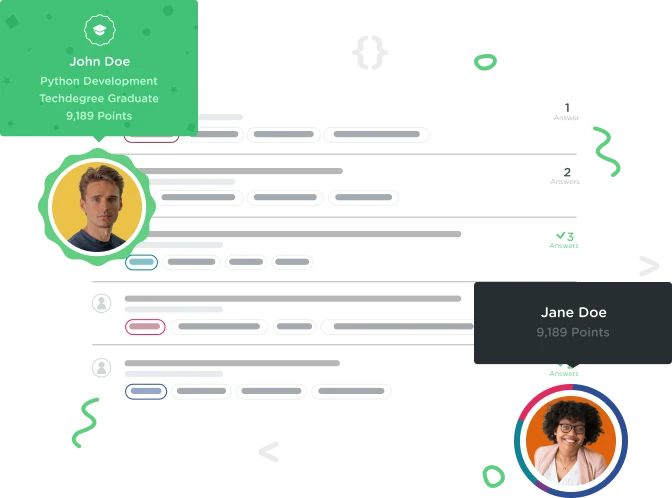

Hanna Reed
3,849 PointsHow can I make a letter my answer for array rather than a number?
I want to make a letter my answer rather than using numbers for my array, so I want A B C D...
Here is my code:
var questions = [
['How many states are in the United States?', 50],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
if (response === answer) { // if the response = the answer, then +1 correctanswer, question you got correct is pushed to the end of the list.
correctAnswers += 1;
correct.push(question);
} else { // if the response doesn't = answer, +1 incorrectanswer, question you got wrong is pushed to the end of the list.
incorrect.push(question);
}
}
2 Answers
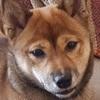
Katie Wood
19,141 PointsHello there,
To make the answer a letter, you just need to put the answer in quotes in the array, like 'A' if the answer is A. Then, you can compare the user response to the answer using ===, just like you can with numbers.
One thing to keep in mind is that, with string answers, you have the option of making your quiz case-sensitive or not. For example, if the answer is 'penguin', and you want to accept 'penguin', 'Penguin', or 'PENGUIN', you would need to compare the all-caps or all-lowercase versions of the answer and the response, like this:
if (response.toUpperCase === answer.toUpperCase)
Hope this helps!

Hanna Reed
3,849 Pointsvar questions = [
['How many states are in the United States? A) 50 B) 49 C)38', 'A'],
['How many continents are there?', 7],
['How many legs does an insect have?', 6]
];
var correctAnswers = 0;
var incorrectAnswers = 0;
var question;
var answer;
var response;
var html;
var correct = [];
var incorrect = [];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function buildList(arr) {
var listHTML = '<ul>';
for (var i = 0; i < arr.length; i += 1) {
listHTML += '<li>' + arr[i] + '</li>';
}
listHTML += '</ul>';
return listHTML;
}
for (var i = 0; i < questions.length; i += 1) {
question = questions[i][0];
answer = questions[i][1];
response = prompt(question);
response = parseInt(response);
if (response === answer) { // if the response = the answer, then +1 correctanswer, question you got correct is pushed to the end of the list.
correctAnswers += 1;
correct.push(question);
} else { // if the response doesn't = answer, +1 incorrectanswer, question you got wrong is pushed to the end of the list.
incorrect.push(question);
}
}
html = "You got " + correctAnswers + " question(s) right. <br>"
html += '<h2 style=" color: #4dffb8; background: #c2d6d6; width: 100%; ">You got these questions correct:</h2>';
html += buildList(correct);
html += '<h2 style=" color: #ff8080; background: #c2d6d6; width: 100%; ">You got these questions incorrect:</h2>';
html += buildList(incorrect);
print(html);
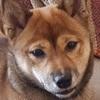
Katie Wood
19,141 PointsOkay, I think I see the problem - if the answer is a string, you don't want to have:
response = parseInt(response);
If you do that, any string value will return NaN. If you just want string answers, you can just take that line out. Or, if you want a mixture of strings and ints as your answers, you can do something like this:
if (isNaN(answer) === false) {
response = parseInt(response);
}
That would make it only parse to int if the response is supposed to be a number.
Let me know how it goes!
Hanna Reed
3,849 PointsHanna Reed
3,849 PointsHmm, not working for me?
Katie Wood
19,141 PointsKatie Wood
19,141 PointsCould you show me what you have now? Maybe I can help :)
Katie Wood
19,141 PointsKatie Wood
19,141 PointsI did notice one thing - I had parentheses where I shouldn't have there, sorry about that! Corrected my answer syntax.