Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial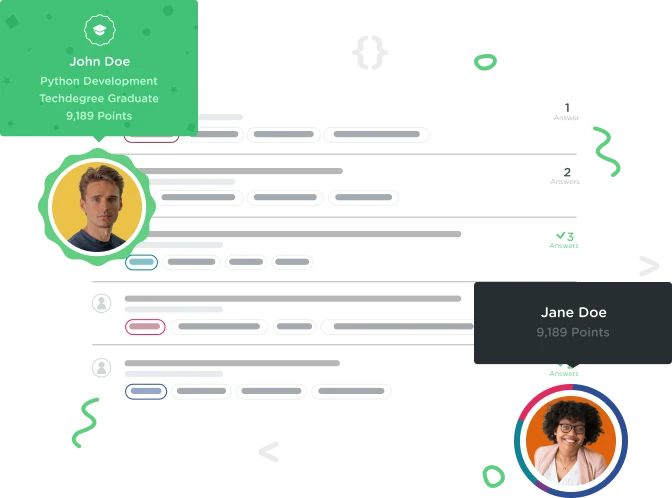

Bryn Clegg
4,698 PointsHow can I make an array of strings of offensive words and use the contains method so i can test for multiple words.
This is my section of code, it apparently contains no errors but the loop doesn't work, if i type in one of the offensive words, the code just continue to on to enter and adverb etc. Here is the relevant section:
boolean isInvalidWord;
String word = "dork toss nerd";
do {
noun = console.readLine("Enter a noun: ");
// Boolean reads back eaither true or false
// use isInvalidWord as a variable for all invalid words to stop repetition
isInvalidWord = (noun.contains(word));
// ban certain language being used in both lower and upper case. \\ means or.
if(isInvalidWord) {
console.printf("That language is not allowed! Try again. \n\n");
}
} while(isInvalidWord);
String adverb = console.readLine("Enter an adverb: ");
String verb = console.readLine("Enter a verb: ");
console.printf("My TreeStoryPrototype:\n---------------\n");
console.printf("%s is a %s %s. They are always %s %s.\n", name, adjective, noun, adverb, verb);
}
}
1 Answer
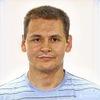
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsChange the line:
isInvalidWord = (noun.contains(word));
To
isInvalidWord = (word.contains(noun));
You are checking that String word
with bad words contains noun
that user has entered.
In your code, you are checking that noun
user entered contains word
, which is not right, so it is like you are checking that word "dork" that you enter contains "dork toss nerd" which will obviously fail...
Bryn Clegg
4,698 PointsBryn Clegg
4,698 PointsCheers that helps a lot. Is there a way of making "dork toss nerd" into some sort of list "dork", "nerd", etc. so that all words that contain those specific words can be banned ie. "tosser"? At the moment i can only test if they put in one of the exact words or a word within one of the longer words eg. "tos"
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsAlexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsI would say you can put your words in a List<String>:
This way only exact match will be checked, i.e.
tos
will not pass, however in your method withString words = "dork toss"
,tos
will be a censored word becauseString words = "dork toss"
containstos
...But that will not solve your problem of finding "tosser", when you set up "toss" as censored word.
In order to remove this particular problem, you can apply additional logic: