Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial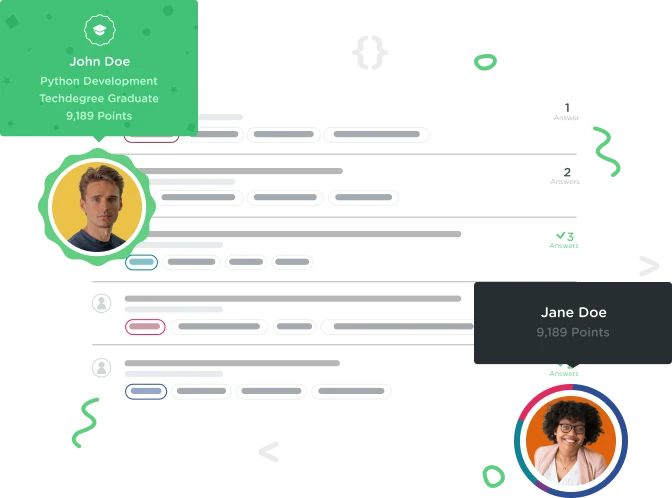

timstrasser2
2,850 PointsHow can i make an Upload System? (php)
How can i let upload users their own pictures? (Like an avatar-pic)
4 Answers
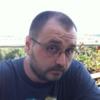
Robert Bojor
Courses Plus Student 29,439 PointsHi Tim,
There are a lot of options when it comes to uploading files on the server. You can do it from a normal form submit, or you can go with AJAX and upload the image in the background while the user continues filling in the form in the page. Personally I like the AJAX way since it doesn't force the user to press the submit button in the form and he can move on once the image is changed.
I am going to show you the normal way since judging by the amount of points in your profile you haven't got too much into JavaScript and AJAX and it will be easier to understand.
You will need two parts of code to achieve the file upload, one is the html form itself and the second one is the actual PHP code that will handle your data. Also, on the server, you will need a folder, for the files to reside in, and the correct permissions for that folder to be set so the web server can write inside of it.
For this example I'm going to use a simple form with just one file element and no other fields. I'm going to assume the page is called index.php and the form will submit its data on this page.
// The form
<form method="post" action="index.php" enctype="multipart/form-data">
<input type="hidden" name="action" id="action" value="uploadFile">
<input type="file" name="avatar" id="avatar">
<button type="submit">Upload avatar</button>
</form>
It is crucial to remember that for a form using file uploads ( AJAX or normal ) you will need to specify the enctype attribute, which specifies how the form-data should be encoded when submitting it to the server. ( You can read more here: http://www.w3schools.com/tags/att_form_enctype.asp )
I have added a hidden field to the form, action, which will allow me to better test the submit and write the conditional in PHP that will handle the upload - I have seen many ways of doing this, I just prefer mine since it's really simple.
When it comes to uploads the actual file data that you have uploaded doesn't come inside the $_POST variable. This data will be available inside the $_FILES variable. ( You can read more on the $_FILES variable here: http://php.net/manual/en/features.file-upload.post-method.php )
// The PHP
if (isset($_POST['action']) && $_POST['action'] == 'uploadFile') {
// Setup a variable containing the path on the server where the uploaded files will reside
// Add the file name to the path
$uploaddir = '/var/www/mysite.com/uploads/';
$uploadfile = $uploaddir.basename($_FILES['avatar']['name']);
// Move the uploaded file to the new location on the server. The move_uploaded_file returns true if successful or false if not, hence the if-else statement
if (move_uploaded_file($_FILES['avatar']['tmp_name'], $uploadfile)) {
echo "File is valid, and was successfully uploaded.";
} else {
echo "Error uploading file!";
}
}
I want to remind you that this is a simple example of how to upload a file to the server and it is better to take this example and improve on it further as you go along and learn more PHP. There are a lot more options to add to this, like form validation, data validation before moving the file to its location, you can also check for a certain file size if you want to have a limit, and so on...
Hope this answered your question and made some things clear. Cheers!

timstrasser2
2,850 PointsThanks for the help! But now i have this problem: Warning: move_uploaded_file(http://localhost/Lovo/upload/image_m.jpg): failed to open stream: HTTP wrapper does not support writeable connections in C:\xampp\htdocs\Lovo\upload.php on line 22
Warning: move_uploaded_file(): Unable to move 'C:\xampp\tmp\php7EAC.tmp' to 'http://localhost/Lovo/upload/image_m.jpg' in C:\xampp\htdocs\Lovo\upload.php on line 22 Error uploading file!
Can you help me?

timstrasser2
2,850 PointsIt works! But only on my webserver, but not on my local xampp server..
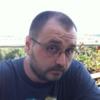
Robert Bojor
Courses Plus Student 29,439 PointsI haven't used windows to code sites in a while, but the errors you are seeing are from the lack of write rights into the final destination folder.
Frankly, I have no idea on how to assign the correct rights on a windows machine. You can check http://stackoverflow.com/questions/8402415/xampp-on-windows-7-set-chmod and http://stackoverflow.com/questions/9349469/changing-php-write-permissions-in-xampp-on-windows-7 to point you in the right direction.