Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial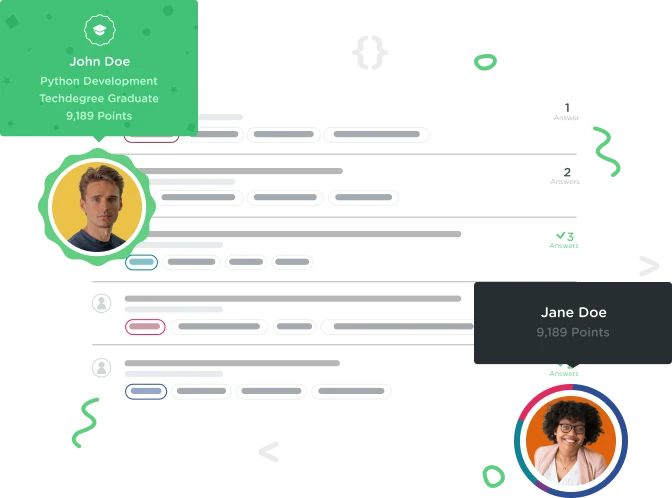
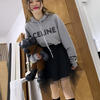
Kevin Narain
11,379 PointsHow can I make it possible that users with hashed passwords can login into my system?
Hi I'm creating a login system where users can log in however, users who don't have a hashed password can login but those who have a hashed password can't login with this system. How can I make it possible that users with a hashed password can also login? Your help is highly appreciated 😊
login.php
<?php
include "db.php";
?>
<?php
session_start(); // Turns on the sessions.
?>
<?php
if (isset($_POST['login'])) {
$username = $_POST['username'];
$password = $_POST['password'];
$username = mysqli_real_escape_string($connection, $username);
$password = mysqli_real_escape_string($connection, $password);
$query = "SELECT * FROM users WHERE username = '{$username}' ";
$select_user_query = mysqli_query($connection, $query);
if (!$select_user_query) {
die("Query failed" . mysqli_error($connection));
}
while($row = mysqli_fetch_assoc($select_user_query)) { //array.
$db_user_id = $row['user_id'];
$db_username = $row['username'];
$db_user_password = $row['user_password'];
$db_user_firstname = $row['user_firstname'];
$db_user_lastname = $row['user_lastname'];
$db_user_role = $row['user_role'];
}
if ($username === $db_username && $password === $db_user_password) {
$_SESSION['username'] = $db_username;
$_SESSION['firstname'] = $db_user_firstname;
$_SESSION['lastname'] = $db_user_lastname;
$_SESSION['user_role'] = $db_user_role;
header("Location: ../admin");
} else {
header("Location: ../index.php");
}
}
?>
registration.php
<?php
include "includes/db.php";
include "includes/header.php";
?>
<?php
if (isset($_POST['submit'])) {
$username = mysqli_real_escape_string($connection, $_POST['username']);
$email = mysqli_real_escape_string($connection, $_POST['email']);
$password = mysqli_real_escape_string($connection, $_POST['password']);
// VALIDATION
if (!empty($username) && !empty($email) && !empty($password)) {
// Hashing
$password = password_hash($password, PASSWORD_DEFAULT);
$query = "INSERT INTO users (username, user_email, user_password, user_role) ";
$query .= "VALUES('{$username}', '{$email}', '{$password}', 'subscriber' )";
$register_user_query = mysqli_query($connection, $query);
if (!$register_user_query) {
die("QUERY FAILED " . mysqli_error($connection) . ' ' . mysqli_errno($connection));
}
$message = "Your registration has been submitted";
} else {
$message = "Fields cannot be empty";
}
} else {
$message = "";
}
?>
<!-- Navigation -->
<?php
include "includes/navigation.php";
?>
<!-- Page Content -->
<div class="container">
<section id="login">
<div class="container">
<div class="row">
<div class="col-xs-6 col-xs-offset-3">
<div class="form-wrap">
<h1>Register</h1>
<form role="form" action="registration.php" method="post" id="login-form" autocomplete="off">
<h6 class="text-center"><?= $message; ?></h6>
<div class="form-group">
<label for="username" class="sr-only">Username</label>
<input type="text" name="username" id="username" class="form-control" placeholder="Enter Desired Username">
</div>
<div class="form-group">
<label for="email" class="sr-only">Email</label>
<input type="email" name="email" id="email" class="form-control" placeholder="somebody@example.com">
</div>
<div class="form-group">
<label for="password" class="sr-only">Password</label>
<input type="password" name="password" id="key" class="form-control" placeholder="Password">
</div>
<input type="submit" name="submit" id="btn-login" class="btn btn-custom btn-lg btn-block" value="Register">
</form>
</div>
</div> <!-- /.col-xs-12 -->
</div> <!-- /.row -->
</div> <!-- /.container -->
</section>
<hr>
<?php include "includes/footer.php";?>
1 Answer

Farai Zuva
6,054 PointsI think you can use the crypt() function which supports several hashing algorithms.