Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial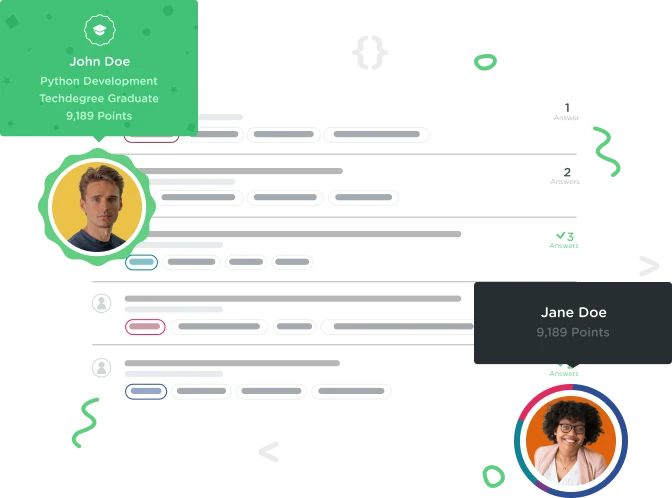

ibbhfsmeaf
4,568 PointsHow can I make my quote transition to other quotes?
If you go to http://desimara.com and look under the main navigation, you will see a quote in green text.
How can I make this quote transition to another quote while keeping all of the properties such as color, background, positioning, etc.?
5 Answers
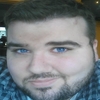
Marcus Parsons
15,719 PointsHey Lansana,
For this, you should set the text to some default text within the HTML DOM, as you have already. Set an id for your paragraph such as "quoteText" so we can directly target that paragraph. Set up some quotes within an array or object to pull from. Then, use some simple JS to switch around the text every so often (let's say at least 9 seconds to give people a chance to read them).
Here is the new div and paragraph
<div id="quoteDiv">
<p id="quoteText">Never believe that a few caring people can't change the world. For, indeed, that's all who ever have.<br><br>- Margaret Mead</p>
</div>
And here is the script.
//Set up the quotes
//Make the very first quote that is actually on the page be the last quote in the array so that it goes full circle
var quotes = [
"The best and most beautiful things in the world cannot be seen or even touched - they must be felt with the heart.<br><br>- Helen Keller",
"Try to be a rainbow in someone's cloud.<br><br>- Maya Angelou",
"Never believe that a few caring people can't change the world. For, indeed, that's all who ever have.<br><br>- Margaret Mead"
];
//Set up a counter variable
var counter = 0;
//Set a timer function, 9000 = 9000 milliseconds = 9 seconds
setInterval(function () {
//If the counter falls within the allowed indices of the array...
if (counter < quotes.length) {
//Set the quote text to the next quote
document.getElementById("quoteText").innerHTML = quotes[counter];
//Increase the counter
counter++;
}
else{
//Otherwise, reset the counter variable
counter = 0;
}
}, 9000);

Greg Kaleka
39,021 PointsHi Lasana,
I would use a combination of these two techniques:
Changing stuff over time with JS
Good luck!

ibbhfsmeaf
4,568 PointsThank you both!
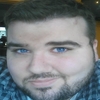
Marcus Parsons
15,719 PointsYou're welcome. Did my code work for you? Check out my comment to my answer, as well.

Greg Kaleka
39,021 PointsNo problem Lasana!
Marcus's code worked flawlessly - you can just copy-paste this into your script file. It's probably also a good idea to check out those two pages on W3Schools so you really understand what's going on, and can apply this to other parts of your site in the future!
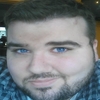
Marcus Parsons
15,719 PointsI agree with Greg 100%, as well. Although I seem to love to hand out answers, it is very important to get to know these concepts yourself. I can include tons of comments, but you'll never truly learn until you put in the work yourself. :)

ibbhfsmeaf
4,568 PointsI actually read through the code and I completely understand whats going on, it's just that I haven't touched JS in a couple of weeks since I have been dealing with HTML/CSS/PHP so I didn't want to have to refresh my brain =P but having read through it all I understand completely what is going on in every line.
Thanks to the both of you! I've updated my site with the code and added some more quotes. I also changed the time.
Also, what do you guys think of my site overall? I'm still working on the email for the forms, I cant seem to get it to email me when I submit despite the fact that my code is done properly - unless of course I'm overlooking something. Maybe you guys knows PHP.
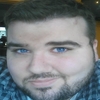
Marcus Parsons
15,719 PointsIt's starting to look really good! If you want to go ahead and post your PHP code, I can take a look at it for you.

ibbhfsmeaf
4,568 PointsHere is the forms.php:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$first_name = $_POST["first_name"];
$last_name = $_POST["last_name"];
$email = $_POST["email"];
$website_description = $_POST["website_description"];
$design_description = $_POST["design_description"];
$email_body = "";
$divider = "----------------------------------------";
$email_body = $email_body . "First Name: " . $first_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Last Name: " . $last_name . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Email: " . $email . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Website Description: " . $website_description . "\n\n" . $divider . "\n\n";
$email_body = $email_body . "Design Description: " . $design_description;
$to = '';
$subject = 'Website Request';
$message = $email_body;
$message = wordwrap($message, 70, "\r\n");
mail($to, $subject, $message);
if(!mail($to, $subject, $message)) {
header("Location: forms.php?status=error");
} else {
header("Location: forms.php?status=complete");
}
}
?>
<?php include('header.php'); ?>
<?php if (isset($_GET["status"]) AND $_GET["status"] == "complete"): ?>
<section id="section-one">
<h2>Your request has been sent! I’ll be in touch shortly.</h2>
</section>
<?php else: ?>
<section id="section-one">
<form method="post" action="index.php">
<fieldset>
<legend><span class="number">1</span>Personal Information</legend>
<label for="first_name" class="required">First Name</label>
<input type="text" id="first_name" name="first_name" required><br>
<label for="last_name">Last Name</label>
<input type="text" id="last_name" name="last_name"><br>
<label for="email" class="required">Email</label>
<input type="email" id="email" name="email" required><br>
</fieldset>
<fieldset>
<legend><span class="number">2</span>Design Information</legend>
<label for="website_description" class="required">Description of Website</label> <p class="labelP">(Do you want a portfolio, informational site, etc.)</p>
<textarea id="website_description" name="website_description" rows="10" cols="60" required></textarea>
<label for="design_description">Description of Design <p class="labelP">(Colors, fonts, etc. If left empty, I will choose.)</p></label>
<textarea id="design_description" name="design_description" rows="10" cols="60"></textarea>
</fieldset>
<hr><br>
<input type="submit" value="Send!" id="finalSubmit"></input>
</form>
</section>
<?php endif; ?>
<?php include('footer.php'); ?>
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsBy the way, a pretty cool way to test this code before putting it on to your site is to load up either Chrome or Firefox, go to your website, and type this into your console (it will show you a live version of this code working without actually changing the code in your website):