Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial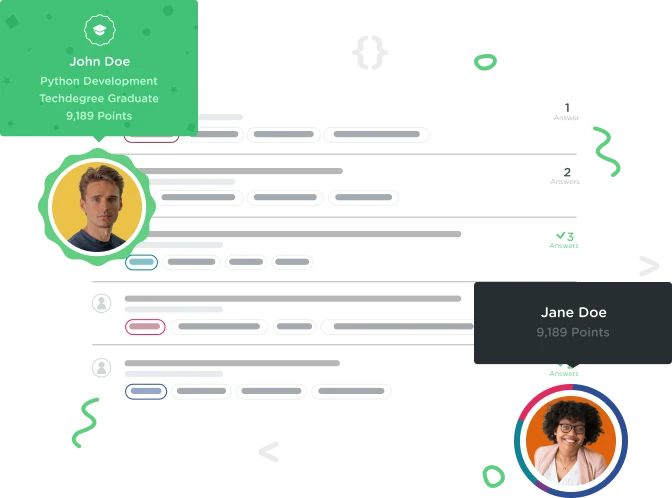
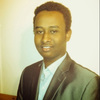
Saleban Olow
Courses Plus Student 141 Pointshow can I make the FreindsFragment Intent?
I am trying to make my FriendsFragment intent to a new class.
For example, When I tap one of my friends, I want to see MessagingFragment class. here is my both classes
FriendsFragment class;
package com.teamtreehouse.ribbit.ui;
import java.util.List;
import android.app.AlertDialog;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.GridView;
import android.widget.TextView;
import com.parse.FindCallback;
import com.parse.ParseException;
import com.parse.ParseQuery;
import com.parse.ParseRelation;
import com.parse.ParseUser;
import com.teamtreehouse.ribbit.R;
import com.teamtreehouse.ribbit.adapters.UserAdapter;
import com.teamtreehouse.ribbit.utils.ParseConstants;
public class FriendsFragment extends Fragment {
public static final String TAG = FriendsFragment.class.getSimpleName();
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected List<ParseUser> mFriends;
protected GridView mGridView;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.user_grid,
container, false);
mGridView = (GridView)rootView.findViewById(R.id.friendsGrid);
TextView emptyTextView = (TextView)rootView.findViewById(android.R.id.empty);
mGridView.setEmptyView(emptyTextView);
return rootView;
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
getActivity().setProgressBarIndeterminateVisibility(true);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, ParseException e) {
getActivity().setProgressBarIndeterminateVisibility(false);
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for(ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
if (mGridView.getAdapter() == null) {
UserAdapter adapter = new UserAdapter(getActivity(), mFriends);
mGridView.setAdapter(adapter);
}
else {
((UserAdapter)mGridView.getAdapter()).refill(mFriends);
}
}
else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(getActivity());
builder.setMessage(e.getMessage())
.setTitle(R.string.error_title)
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
MessagingFragment class,
package com.teamtreehouse.ribbit.ui;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import android.content.Context;
import android.os.Bundle;
import android.os.Handler;
import android.text.InputType;
import android.text.format.DateUtils;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.view.inputmethod.InputMethodManager;
import android.widget.AbsListView;
import android.widget.BaseAdapter;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
import com.parse.FindCallback;
import com.parse.ParseAnalytics;
import com.parse.ParseException;
import com.parse.ParseObject;
import com.parse.ParseQuery;
import com.parse.ParseUser;
import com.parse.SaveCallback;
import com.teamtreehouse.ribbit.R;
import com.teamtreehouse.ribbit.custom.CustomActivity;
import com.teamtreehouse.ribbit.model.Conversation;
import com.teamtreehouse.ribbit.utils.Const;
public class Chat extends CustomActivity
{
public static ParseUser Username;
/** The Conversation list. */
private ArrayList<Conversation> convList;
/** The chat adapter. */
private ChatAdapter adp;
/** The Editext to compose the message. */
private EditText txt;
/** The user name of buddy. */
private String buddy;
/** The date of last message in conversation. */
private Date lastMsgDate;
/** Flag to hold if the activity is running or not. */
private boolean isRunning;
/** The handler. */
private static Handler handler;
/* (non-Javadoc)
* @see android.support.v4.app.FragmentActivity#onCreate(android.os.Bundle)
*/
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.chat);
ParseAnalytics.trackAppOpened(getIntent());
convList = new ArrayList<Conversation>();
ListView list = (ListView) findViewById(R.id.list);
adp = new ChatAdapter();
list.setAdapter(adp);
list.setTranscriptMode(AbsListView.TRANSCRIPT_MODE_ALWAYS_SCROLL);
list.setStackFromBottom(true);
txt = (EditText) findViewById(R.id.txt);
txt.setInputType(InputType.TYPE_CLASS_TEXT
| InputType.TYPE_TEXT_FLAG_MULTI_LINE);
setTouchNClick(R.id.btnSend);
buddy = getIntent().getStringExtra(Const.EXTRA_DATA);
getActionBar().setTitle(buddy);
handler = new Handler();
}
/* (non-Javadoc)
* @see android.support.v4.app.FragmentActivity#onResume()
*/
@Override
protected void onResume()
{
super.onResume();
isRunning = true;
loadConversationList();
}
/* (non-Javadoc)
* @see android.support.v4.app.FragmentActivity#onPause()
*/
@Override
protected void onPause()
{
super.onPause();
isRunning = false;
}
/* (non-Javadoc)
* @see com.socialshare.custom.CustomFragment#onClick(android.view.View)
*/
@Override
public void onClick(View v)
{
super.onClick(v);
if (v.getId() == R.id.btnSend)
{
sendMessage();
}
}
/**
* Call this method to Send message to opponent. It does nothing if the text
* is empty otherwise it creates a Parse object for Chat message and send it
* to Parse server.
*/
private void sendMessage()
{
if (txt.length() == 0)
return;
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
imm.hideSoftInputFromWindow(txt.getWindowToken(), 0);
String s = txt.getText().toString();
final Conversation c = new Conversation(s, new Date(),
UserListActivity.Username.getUsername());
c.setStatus(Conversation.STATUS_SENDING);
convList.add(c);
adp.notifyDataSetChanged();
txt.setText(null);
ParseObject po = new ParseObject("Chat");
po.put("sender", UserListActivity.Username.getUsername());
po.put("receiver", buddy);
// po.put("createdAt", "");
po.put("message", s);
po.saveEventually(new SaveCallback() {
@Override
public void done(ParseException e)
{
if (e == null)
c.setStatus(Conversation.STATUS_SENT);
else
c.setStatus(Conversation.STATUS_FAILED);
adp.notifyDataSetChanged();
}
});
}
/**
* Load the conversation list from Parse server and save the date of last
* message that will be used to load only recent new messages
*/
private void loadConversationList()
{
ParseQuery<ParseObject> q = ParseQuery.getQuery("Chat");
if (convList.size() == 0)
{
// load all messages...
ArrayList<String> al = new ArrayList<String>();
al.add(buddy);
al.add(UserListActivity.Username.getUsername());
q.whereContainedIn("sender", al);
q.whereContainedIn("receiver", al);
}
else
{
// load only newly received message..
if (lastMsgDate != null)
q.whereGreaterThan("createdAt", lastMsgDate);
q.whereEqualTo("sender", buddy);
q.whereEqualTo("receiver", UserListActivity.Username.getUsername());
}
q.orderByDescending("createdAt");
q.setLimit(30);
q.findInBackground(new FindCallback<ParseObject>() {
@Override
public void done(List<ParseObject> li, ParseException e)
{
if (li != null && li.size() > 0)
{
for (int i = li.size() - 1; i >= 0; i--)
{
ParseObject po = li.get(i);
Conversation c = new Conversation(po
.getString("message"), po.getCreatedAt(), po
.getString("sender"));
convList.add(c);
if (lastMsgDate == null
|| lastMsgDate.before(c.getDate()))
lastMsgDate = c.getDate();
adp.notifyDataSetChanged();
}
}
handler.postDelayed(new Runnable() {
public void run()
{
if (isRunning)
loadConversationList();
}
}, 1000);
}
});
}
/**
* The Class ChatAdapter is the adapter class for Chat ListView. This
* adapter shows the Sent or Receieved Chat message in each list item.
*/
private class ChatAdapter extends BaseAdapter
{
/* (non-Javadoc)
* @see android.widget.Adapter#getCount()
*/
public int getCount()
{
return convList.size();
}
/* (non-Javadoc)
* @see android.widget.Adapter#getItem(int)
*/
public Conversation getItem(int arg0)
{
return convList.get(arg0);
}
/* (non-Javadoc)
* @see android.widget.Adapter#getItemId(int)
*/
public long getItemId(int arg0)
{
return arg0;
}
/* (non-Javadoc)
* @see android.widget.Adapter#getView(int, android.view.View, android.view.ViewGroup)
*/
public View getView(int pos, View v, ViewGroup arg2)
{
Conversation c = getItem(pos);
if (c.isSent())
v = getLayoutInflater().inflate(R.layout.chat_item_sent, null);
else
v = getLayoutInflater().inflate(R.layout.chat_item_rcv, null);
TextView lbl = (TextView) v.findViewById(R.id.lbl1);
lbl.setText(DateUtils.getRelativeDateTimeString(Chat.this, c
.getDate().getTime(), DateUtils.SECOND_IN_MILLIS,
DateUtils.DAY_IN_MILLIS, 0));
lbl = (TextView) v.findViewById(R.id.lbl2);
lbl.setText(c.getMsg());
lbl = (TextView) v.findViewById(R.id.lbl3);
if (c.isSent())
{
if (c.getStatus() == Conversation.STATUS_SENT)
lbl.setText("Delivered");
else if (c.getStatus() == Conversation.STATUS_SENDING)
lbl.setText("Sending...");
else
lbl.setText("Failed");
}
else
lbl.setText("");
return v;
}
}
/* (non-Javadoc)
* @see android.app.Activity#onOptionsItemSelected(android.view.MenuItem)
*/
@Override
public boolean onOptionsItemSelected(MenuItem item)
{
if (item.getItemId() == android.R.id.home)
{
finish();
}
return super.onOptionsItemSelected(item);
}
}
1 Answer

Danial Goodwin
13,247 PointsIf you tried navigating to a Fragment
with something like startActivity()
, then you'll notice an error because it's not an Activity
.
There's two main ways to show a Fragment. Either you can put the Fragment in another Activity's layout XML and just call startActivity()
to that containing Activity. Or, you can dynamically load a Fragment in the current Activity.
To navigate to a Fragment dynamically from within an Activity, here's some sample code:
public void navigateToFriendsFragment() {
getFragmentManager().beginTransaction()
.add(R.id.container, new FriendsFragment())
.commit();
}
The R.id.container
is a special id that refers to the entire screen. There are plenty of options that can be added to this FragmentManager
and but this should get you started. I'd highly recommend reading more about Fragments
to find out some more great things you can do: http://developer.android.com/guide/components/fragments.html
Sidenote: Depending on which Fragment
API you are using, you might need to use getSupportFragmentManage()
instead.
Danial Goodwin
13,247 PointsDanial Goodwin
13,247 PointsSomething I would liked to have seen in the question is what you tried and what error message you had. Having the more straight-forward question and code and what you have already tried typically allows others to potentially provide better answers, quicker and easier.