Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial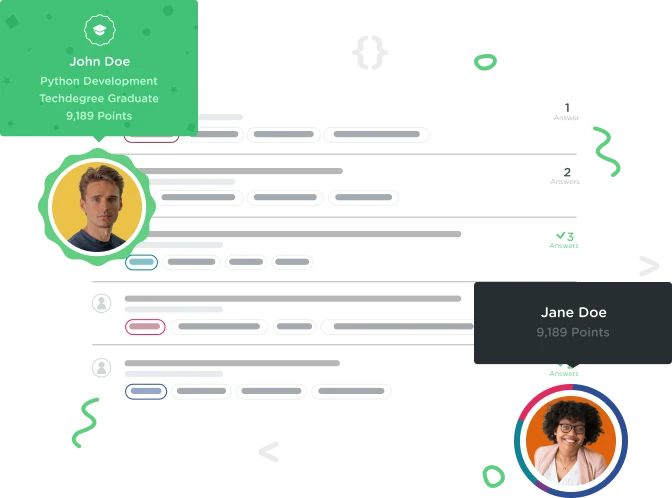
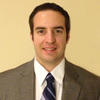
Anthony Domina
Courses Plus Student 19,571 PointsHow can I make this jQuery more efficient?
The issue is on my website at the link below:
http://be-tony.com/JapanPrefectureMap.html
What I have is an SVG of Japan. Each prefecture is already identified and has a class which is represented in my CSS.
In my HTML I have an unordered list and so far I have only given ID's to two prefectures: Aichi and Hyogo.
The jQuery script below allows me to hover over one of these two prefectures in the unordered list and in the map the color goes from lightgreen to purple while in the hover state.
Now, I could do a hover function for every prefecture but that seems tedious. Here is the endgame I could use some help with:
The classes in my CSS will match the name of the IDs in the unordered list, for example .hyogoKen and #hyogoKen.
How can I craft a jQuery statement/condition that allows me to say if ClassName equal to ID name? The goal is to have a universal Jquery statement that allows me to hover over a list item and then it finds in the map the item with the same name.
Any help is appreciated! Thank you!
MY HTML
<div class="col-md-3 pull-right offset-1">
<ul id="JapanMapList" class="jml">
<li id="aichiKen">Aichi</li>
<li>Fukuoka</li>
<li>Hiroshima</li>
<li id="hyogoKen">Hyogo</li>
<li>Kagoshima</li>
<li>Kanagawa</li>
<li>Kumamoto</li>
<li>Kyoto</li>
<li>Nagano</li>
<li>Nara</li>
<li>Oita</li>
<li>Osaka</li>
<li>Tochigi</li>
<li>Tokyo</li>
</ul>
</div>
<script>
$(document).ready(function(){
$(".jml #hyogoKen").hover(
function()
{
$(this).css("background-color", "lightgreen");
$(".hyogoKen").css("animation", "none");
$(".hyogoKen").css("fill", "purple");
},
function() {
$(this).css("background-color", "#FFF");
$(".beenHere").css("fill", "lightgreen");
}
);
$(".jml #aichiKen").hover(
function()
{
$(this).css("background-color", "lightgreen");
$(".aichiKen").css("animation", "none");
$(".aichiKen").css("fill", "purple");
},
function() {
$(this).css("background-color", "#FFF");
$(".beenHere").css("fill", "lightgreen");
}
);
});
</script>
MY CSS
@keyframes fill-lightgreen {
100% {
fill: lightgreen;
stroke: black;
}
}
.kumamotoKen {
animation: fill-lightgreen .5s 1s forwards;
}
.kyotoKen {
animation: fill-lightgreen .5s 2s forwards;
}
.tokyoKen {
animation: fill-lightgreen .5s 3s forwards;
}
.kagoshimaKen {
animation: fill-lightgreen .5s 4s forwards;
}
.kanagawaKen {
animation: fill-lightgreen .5s 5s forwards;
}
.hiroshimaKen {
animation: fill-lightgreen .5s 6s forwards;
}
.naganoKen {
animation: fill-lightgreen .5s 7s forwards;
}
.fukuokaKen {
animation: fill-lightgreen .5s 8s forwards;
}
.osakaKen {
animation: fill-lightgreen .5s 9s forwards;
}
.tochigiKen {
animation: fill-lightgreen .5s 10s forwards;
}
.aichiKen {
animation: fill-lightgreen .5s 11s forwards;
}
.oitaKen {
animation: fill-lightgreen .5s 12s forwards;
}
.naraKen {
animation: fill-lightgreen .5s 13s forwards;
}
.hyogoKen {
animation: fill-lightgreen .5s 14s forwards;
}
#JapanMapList {
margin-top: 50px;
font-size: 18pt;
color: darkgreen;
}
4 Answers
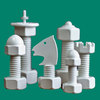
Steven Parker
230,274 PointsYou don't even need to give ID's to the list items.
As long as the class name of the SVC region is consistently the name of the prefecture in lower case followed by "Ken", this replacement block should handle all of them:
$(".jml li").hover(
function() {
$(this).css("background-color", "lightgreen");
var prefectureClass = "." + $(this).html().toLowerCase() + "Ken";
$(prefectureClass).css("animation", "none").css("fill", "purple");
},
function() {
$(this).css("background-color", "#FFF");
$(".beenHere").css("fill", "lightgreen");
}
);
And, very cool project!

Carrie Short
3,529 PointsYou can retrieve the id of the li and then store it in a variable and target the class using concatination
$(".jml li").hover(
function()
{
var location = $(this).attr('id');
$("." + location).css("fill", "purple");
}
);
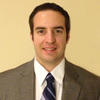
Anthony Domina
Courses Plus Student 19,571 PointsGreat suggestions! I can't wait to try them out! Thank you both very much for your input!
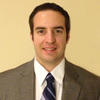
Anthony Domina
Courses Plus Student 19,571 PointsTried Steven's solution first and it worked flawlessly! Even added a few css extras to color things up a little bit!
$(".jml li").hover(
function() {
$(this).css("background-color", "lightgreen");
$(this).css("color", "purple");
var prefectureClass = "." + $(this).html().toLowerCase() + "Ken";
$(prefectureClass).css("animation", "none").css("fill", "purple");
},
function() {
$(this).css("background-color", "#FFF");
$(this).css("color", "darkgreen");
$(".beenHere").css("fill", "lightgreen");
}
);
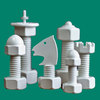
Steven Parker
230,274 PointsVery cool again. And remember, you can chain those .css calls if you want.