Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial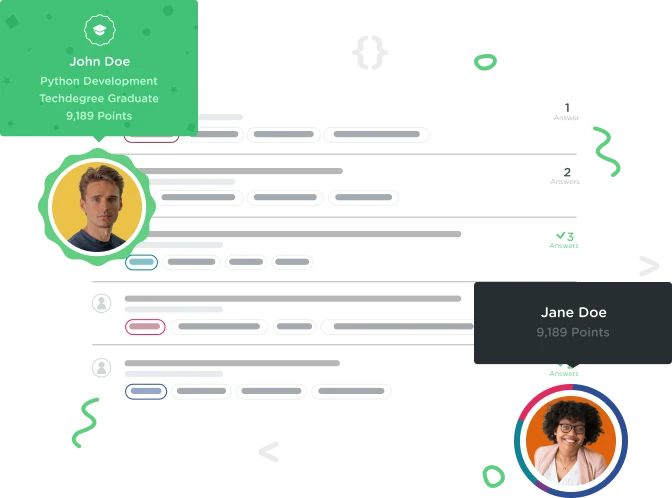

Mark Libario
9,003 PointsHow can I print different numbers per DIV?
Guys need help!
Im already in the objects course but Im going back to review and apply these stuff.
What I wanted to do is to imitate a lottery draw.
I created an object that holds a lottery number and holds a function that draws that number randomly from 1 - 49.
I wanted the page to print out my 6 divs that contains 6 different numbers from the lottery object.
However, idk how to do it. I just end up printing 6 numbers and all the same numbers..
can anyone show me how to do it right?
Im thinking of an array or something like adding a new property on the object for each draw and then prints out all the numbers in the array or in the object, something like this.
Here is my JavaScript:
function lotto (number) {
this.number = number;
this.drawNumber = function () {
return Math.floor(Math.random() * number) +1;
}
}
var lottoNumber = new lotto (49);
var draw = lottoNumber.drawNumber ();
let html = "";
for (let i = 0 ; i < 6; i+= 1) {
html += "<div>" + draw + "</div>";
}
document.write (html);
2 Answers
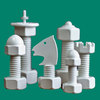
Steven Parker
231,261 PointsYou're getting the same number because you only pick a random number once before the loop, and then just print the same number over and over in the loop. You can change this by picking the random number inside the loop:
html += "<div>" + lottoNumber.drawNumber() + "</div>";
But even this might not be what you really want, since sometimes you will get the same number chosen randomly more than once but lotto numbers must all be unique. To accomplish this, you might want to pick the numbers much like they do in real life using the ping-pong balls. Your "balls" could be an array with all the numbers, and for each pick you could select one at random and then remove it from the array. So every choice would be made from a slightly smaller set.
Also, by convention constructor function names start with a capital letter.
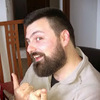
Marco Poletto
Courses Plus Student 10,099 PointsYou should run the random number generator inside the for loop. Something like that
for (let i = 0 ; i < 6; i+= 1) {
let numberLoop = lotto(49);
html += "<div>" + numberLoop + "</div>";
}
I haven't tested it but it should work
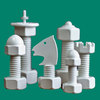
Steven Parker
231,261 PointsNote that "lotto" is a constructor function and doesn't return anything.
Mark Libario
9,003 PointsMark Libario
9,003 Pointsbut by doing lottoNumber.drawNumber () , isnt it the same thing as I did because i placed that function in the variable named draw. so instead of typing the whole thing, al i need to do is typed draw that calls that function.. I dont get it :/
Steven Parker
231,261 PointsSteven Parker
231,261 PointsWhat the original code does is invoke (same thing as "call") the function, and put the result (the random number it picked) in the variable named "draw". So "draw" represents the number, not the function itself.
Mark Libario
9,003 PointsMark Libario
9,003 PointsOh i see, that's true! I totally forgot about that. it's like naming a variable, and then using prompt. Thanks!
I tried to put the same like you mentioned, however it gives me this:
function () { return Math.floor(Math.random() * number) +1; }function () { return Math.floor(Math.random() * number) +1; }function () { return Math.floor(Math.random() * number) +1; }function () { return Math.floor(Math.random() * number) +1; }function () { return Math.floor(Math.random() * number) +1; }function () { return Math.floor(Math.random() * number) +1; }
its like repetitive of what is inside of the this,drawNumber and not giving a number instead.
Im using this Workspace for reference: https://teamtreehouse.com/library/for-loops-2
I am just working on the javascript side.
Mark Libario
9,003 PointsMark Libario
9,003 PointsI got it now!
I did something like this.
Steven Parker
231,261 PointsSteven Parker
231,261 PointsSure, that would work, but it's a bit unusual:
You might do the same thing a bit more conventionally (and compactly) like this: