Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial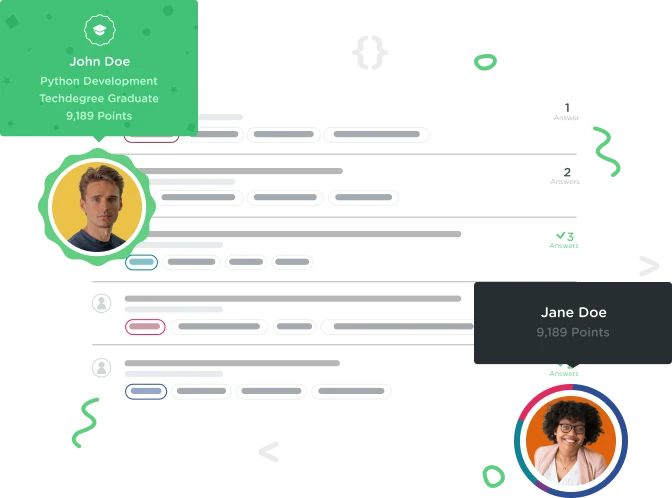

Mark Conway
1,215 PointsHow can I retrieve the value from an input using jQuery?
I'm trying to get past a code challenge in Treehouse. It's asking me to retrieve a value for the text input so that it can be displayed as a h1. Can anyone shed some light on how I might do this? I'm just a beginner at Js. Thanks
//Select the input for the title for blog post.
var $titleInput = $("#title");
//Select the preview h1 tag
var $previewTitle = $("#titlePreview");
// Every second update the preview
var previewTimer = setInterval(updatePreview, 1000);
function updatePreview(){
//Get the user's input
var titleValue =
//Set the user input as the preview title.
$previewTitle.text(titleValue);
}
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" type="text/css" href="theme.css">
</head>
<body>
<div id="form">
<label for="title">Blog Post Title</label><input id="title" name="title" value="titleValue" placeholder="Enter your blog title here">
</div>
<div id="preview">
<h1 id="titlePreview"></h1>
</div>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
#form {
width: 45%;
float: left;
}
#preview {
width: 45%;
float: right;
}
label {
width: 20%;
display: inline-block;
}
input {
width: 70%;
}
3 Answers

Petros Sordinas
16,181 PointsHi Mark,
var titleValue = $('#title').val();
$('#title') is the jquery selector for the element with an id of 'title' (your input element), val() is the method to retrieve the value of the element.

Jacob Mishkin
23,117 PointsIt's totally cool, this is a three prong challenge. The question is asking you to retrieve the value of the user input and place its value in the variable $titleValue. The first thing is to look at the HTML and find the form that users will be inputting data.. In the Jquery file there is a variable set called $titleInput and has the corresponding id tag:
//Select the input for the title for blog post.
var $titleInput = $("#title");
So now what we need to do is take that variable and have it equal the variable $titleValue like so:
function updatePreview(){
//Get the user's input
var titleValue = $titleInput.val();
//Set the user input as the preview title.
$previewTitle.text(titleValue);
So you are taking the input that is in the form and then placing the value into the variable titleValue.

Mark Conway
1,215 PointsHi Jacob and Petros! Thanks very much for both of your answers! Very informative and well explained! I got it to work in the end and understand it a lot more now! Thanks again!

Jacob Mishkin
23,117 PointsNot a problem, We are here to help.