Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial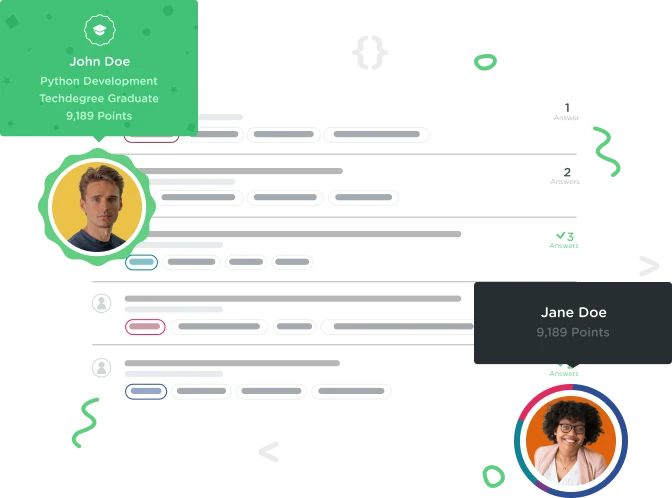

Arsalan Raja
6,508 PointsHow can i search specific name in my array?
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var reaponse;
var html;
function print(message) {
document.write(message);
}
for(var i = 0; i < students.length; i++) {
var studentName = students[i].name;
var studentTrack = students[i].track;
var studentAchievements = students[i].achievements;
var studentPoints = students[i].points;
response = prompt("Enter student name? or type 'quit' for exit");
if( response === "quit"){
break;
}
else if(response === studentName) {
html = "<h2> " +studentName+ "</h2>";4
html += "<p>Track: " + studentTrack + "</p>";
html += "<p>Achievements: " + studentAchievements + "</p>";
html += "<p>Points: " + studentPoints + "</p>";
}
else if(response !== studentName) {
html = "Not found";
}
print(html);
}
Edited to display the markdown correctly. :-)
2 Answers
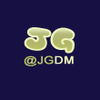
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi there,
It looks like you have a typo when you're declaring your response variable for the first time. Change your variable just under the students object from reaponse to response
Good luck. :-)

Iain Simmons
Treehouse Moderator 32,305 PointsThis won't really work as a search, because you're showing a prompt in each iteration of the loop, and it will only work if the response matches the student at the current index.
Instead, you should use a while
loop to show the prompt, check for quit
, and then if a name is typed in, loop through the students
array and check for each item in the array if that item has a name
property that matches the response
.
Like so:
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: 175,
points: 16375
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: 55,
points: 2025
},
{
name: 'John',
track: 'Learn WordPress',
achievements: 40,
points: 1950
},
{
name: 'Trish',
track: 'Rails Development',
achievements: 5,
points: 350
}
];
var response;
var html;
var found = false;
var studentName;
var studentTrack;
var studentAchievements;
var studentPoints;
var i;
function print(message) {
document.write(message);
}
// loop continuously until found is set to true or the user quits
while (!found) {
response = prompt("Enter student name or type 'quit' for exit");
if (response.toLowerCase() === "quit") {
// break out of the while loop
break;
}
else {
// initially set html to not found,
// and only change if something matches
html = "Not found";
for(i = 0; i < students.length; i++) {
studentName = students[i].name;
studentTrack = students[i].track;
studentAchievements = students[i].achievements;
studentPoints = students[i].points;
// convert to lowercase so they don't need
// to get the capitalisation correct
if (response.toLowerCase() === studentName.toLowerCase()) {
// set found to true to stop the while loop
found = true;
// set the html output
html = "<h2>" + studentName + "</h2>";
html += "<p>Track: " + studentTrack + "</p>";
html += "<p>Achievements: " + studentAchievements + "</p>";
html += "<p>Points: " + studentPoints + "</p>";
// break out of the for loop
break;
}
// you don't want an else in the for loop,
// because you don't want to do something for
// every item that doesn't match
}
}
// print either the not found message or the matching student
print(html);
}