Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial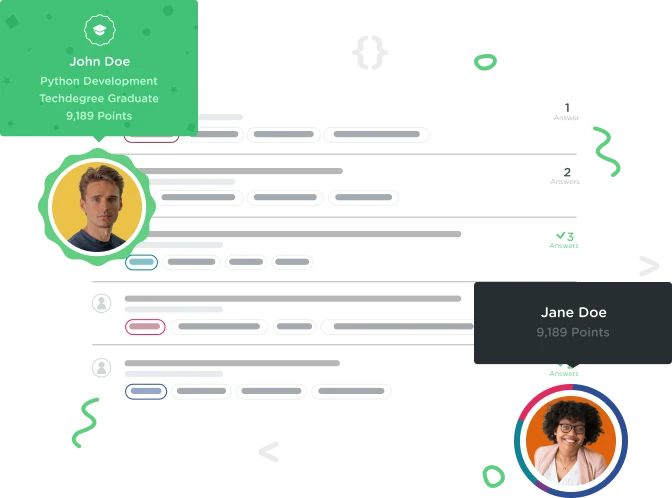

Dario Hunt
2,245 PointsHow can I separate the edit registration page created by Devise?
My current edit registration page is becoming too long and I want to be able to split this into two separate pages. So for example, all the account information (password, email, etc.) would be at site.com/settings and the profile fields (username, bio, etc.) would continue to be at site.com/edit
I haven't been able to find any good documentation on this. Anyone know how to accomplish this?
3 Answers
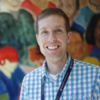
Brandon Barrette
20,485 PointsI would personally make them separate models. Devise for registration and user sessions, and a model called user_settings for all the other stuff. Then you could easily route each of those pages in your routes file.
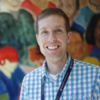
Brandon Barrette
20,485 PointsYeah, this is why i would just create a new model and controller for user_settings. There should be no new action (you could set it to automatically create a row in the database on signup) and only be an edit/update action. That way you could just call:
@settings = current_user.user_settings
Then in the edit form you can just reference @settings.

Dario Hunt
2,245 PointsAlright I've figured this one and I didn't have to create a separate model, trying to keep everything simple for future integration. I'll post my answer for anyone else who may run into this problem. Surprised there wasn't clearer documentation on this.
Create a separate RegistrationsController that inherits from Devise's generated controller:
class RegistrationsController < Devise::RegistrationsController
def settings
@member = current_member
if @member
render :settings
else
render file: 'public/404', status: 404, formats: [:html]
end
end
end
Create the corresponding view in your Devise registrations folder (I just copied the fields I needed from the existing edit form) and then call to the method in your routes:
devise_scope :member do
root :to => 'devise/registrations#new'
match '/settings' => 'registrations#settings', as: :settings
end
Only thing I need to figure out is how to require a password on one form, but not the other.

Garrett Goehring
7,884 PointsI tried this, however I get an error saying that it does not recognize resource_name
Dario Hunt
2,245 PointsDario Hunt
2,245 PointsHm, you think it'd be possible to just create another registration controller that inherits from Devise then define a settings method and do the proper routing? Something like:
I think I'm gonna give that a go, I'll let you know how that goes lol.
Dario Hunt
2,245 PointsDario Hunt
2,245 PointsOk I was almost right. I was able to get it to recognize the page and method by including this in the new RegistationsController:
and this in my routes:
However, I need to do something with that @member so the form pulls in any pre-existing information the member already has. It'd be easier if I could actually look at what's in the Devise Controller.