Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial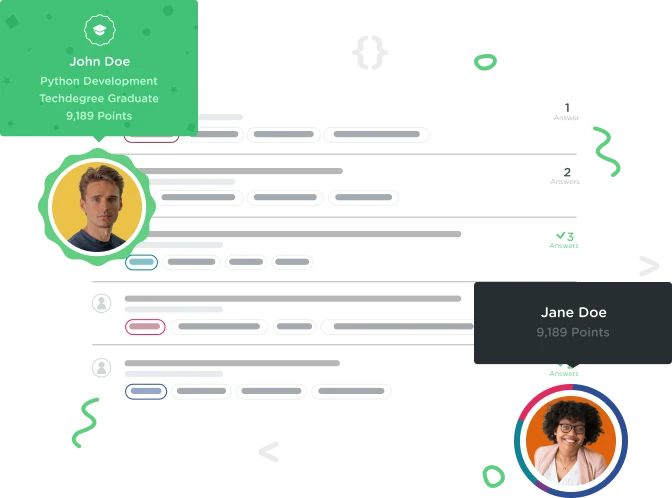

J V
3,414 PointsHow can I set the first alert box to appear 5 seconds after the user has opened the webpage?
Here is my code. I am just looking for any advice or tips that may be helpful for the future.
/***********************
MY PROGRAM
***********************/
document.write("Before you being, please open the console log in Google Chrome. Once you have the console log open, please click the console tab."
+ "You can begin now." + "<br>");
var question1 = prompt("Enter your name: ");
var question2 = prompt("Enter your age: ");
function userDetails(name,age)
{
/*NULL is represent a value that is not known*/
/*Empty means that the variable is empty*/
if(question1 === null || question1 =='')
{
throw new Error("The name field needs to be filled out. " +
"JavaScript lets you create your own errors, which print directly "
+ "to the JavaScript console, just like regular syntax errors do.");
}
else if (question1.length <= 2)
{
throw new Error("The name needs to be longer than 2 characters");
}
else
{
document.write("Name: " + name + "<br>");
document.write("Age: " + age + "<br>");
}
}
userDetails(question1, question2);
/*****************************************************************
******************************************************************/
document.write("Since we have your information, we can now play a quick game." + "<br>" + "<br>");
var question3 = prompt("Enter the number of days you work per week: ");
if(question3 <=7)
{
document.write("Days per week working: " + question3 + "<br>")
}
else if(question3 >= 8)
{
alert("INVALID! There are not " + question3 + " days in the week. ");
var question3 = prompt("Enter the number of days you work per week: ");
if(question3 <= 7)
{
document.write("Days per week working: " + question3 + "<br>");
}
else
{
throw new Error("Something went terribly wrong! There are only 7 days in a week.");
}
}
/*****************************************************************
******************************************************************/
var question4 = prompt("Enter the number of hours you work per day: ");
document.write("Hours per day: " + question4 + "<br>");
/*****************************************************************
******************************************************************/
var weekly_total = (question3 * question4);
document.write("Hours per week: " + weekly_total + "<br>");
alert("Since we now have the number of hours that you work per week, you will be asked to choose a minimum number." +
"The number you choose will be used to generate a random number between the number of hours per week (Maximum Value) and your value (The Minimum Value).");
/*****************************************************************
******************************************************************/
var questionMin = parseInt(prompt("Please enter a minimum value please: "));
if(questionMin >= weekly_total)
{
alert("This is not possible. The minimum value cannot be greater than: " + weekly_total);
var questionMin = parseInt(prompt("Please enter a minimum value please: "));
if(questionMin < weekly_total)
{
function randomGuess(min, max)
{
var randomNumber = Math.floor(Math.random() * (max-min + 1)) + min;
document.write("Maximum Value: " + weekly_total + "<br>");
document.write("Minimum Value: " + questionMin + "<br>");
document.write("The random number is: " + randomNumber + "<br>");
return randomNumber;
}
}
else
{
throw new Error("Booyah! Something went terribly wrong!");
}
}
randomGuess(questionMin, weekly_total);
1 Answer

Mikkel Rasmussen
31,772 PointsIf you have jquery included - can you do it with this:
setTimeout(function() {
// Things to do after 5 seconds
}, 5000);
Colin Bell
29,679 PointsColin Bell
29,679 PointssetTimeout()
is a plain JavaScript function. You don't need jQuery for it.