Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial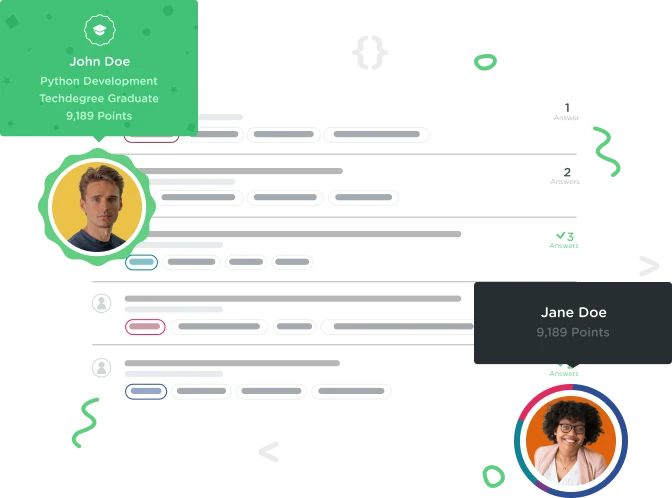

Miles Grofsorean
1,345 PointsHow can I solve FizzBuzz with switch statements?
When I write out my cases, Xcode tells me "expression pattern of type 'Bool' cannot match values of type 'Int'". Why is this the case? I haven't declared any true/false constants, and I'm not sure how to declare what type (string, bool, int, etc) each case should produce (not sure that's even possible).
func fizzBuzz(n: Int) -> String {
switch n {
case n % 3 == 0: print("Fizz")
case n % 5 == 0: print("Buzz")
case n % 3 == 0 && n % 5 == 0: print("FizzBuzz")
default: print ("\(n)")
}
return "\(n)"
}
3 Answers
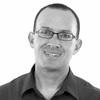
David Papandrew
8,386 PointsHey Miles, I don't know if this is the optimal way to do it, but this is how I passed this challenge:
func fizzBuzz(n: Int) -> String {
switch (n%3, n%5) {
case (0, 0): return "FizzBuzz"
case (0, 1..<n): return "Fizz"
case (1..<n, 0): return "Buzz"
default: return "\(n)"
}
}

Muratalin Appas
1,725 PointsYou need to use "true" or "false" on switch statement and also you need to return something (like Strings: "Fizz", "Buzz") after each case.
func fizzBuzz(n: Int) -> String {
// Enter your code between the two comment markers
switch true {
case n % 3 == 0 && n % 5 == 0: return "FizzBuzz"
case n % 3 == 0: return "Fizz"
case n % 5 == 0: return "Buzz"
default: print(n)
}
// End code
return "\(n)"
}
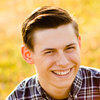
Caleb Kleveter
Treehouse Moderator 37,862 PointsThe reason you get this is because when you have a statement with an equality operator ==
, the statement returns true or false. That is the way programming languages work.