Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial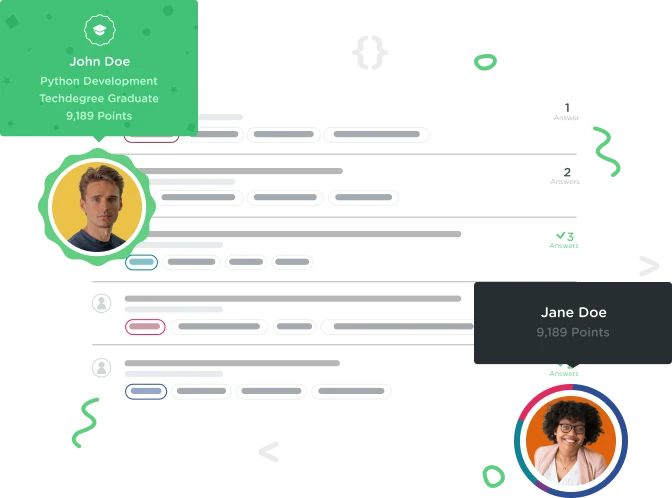
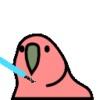
Vincent Nguyen
4,520 PointsHow can I store these in the times5 array?
I need help storing the numbers in the times5 array.
const numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// times5 should be: [5,10,15,20,25,30,35,40,45,50]
// Write your code below
function multiply(numbers){
return numbers * 5;
}
3 Answers

Jackson Monk
4,527 Pointsconst numbers = [1,2,3,4,5,6,7,8,9,10];
let times5 = [];
// times5 should be: [5,10,15,20,25,30,35,40,45,50]
// Write your code below
numbers.forEach(function(currentNum) {
times5.push(currentNum * 5);
});
This way, using forEach, you can iterate through each number and add it to times5
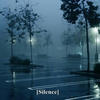
Thayer Y
29,789 Pointsit's just another way to pass the challenge.
numbers.forEach(number => times5.push(number * 5));

Cooper Runstein
11,850 PointsJackson is technically right, though forEach is not typically a best practice when working with arrays, it's quite slow when compared to a for loop, and is generally not a good idea if you can avoid it when working with array and array like objects. If you want to use a built in method, map is a better choice when working with arrays. The course you're working on probably wants you to do something like this:
for (let i = 0; i < numbers.length; i++){
times5.push(numbers[i] * 5)
}
The quickest way to do it in terms of code written is something like:
times5 = numbers.map(num => num*5)
If the second one doesn't make any sense yet, that's fine, in terms of speed and efficiency, the for loop is probably your best bet. If you're comfortable with the second solution, just remember it will only work if you declare times5 with let.
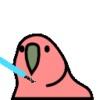
Vincent Nguyen
4,520 PointsThank you for helping! :)
Vincent Nguyen
4,520 PointsVincent Nguyen
4,520 PointsThank you for helping! :)