Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial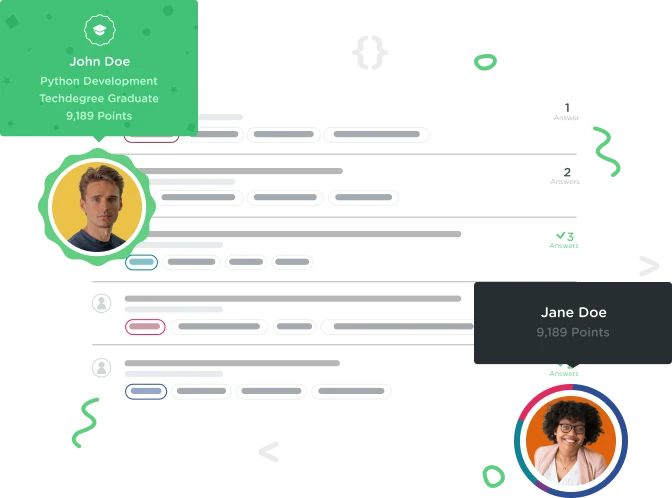
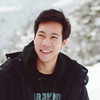
Howie Yeo
Courses Plus Student 3,639 PointsHow can i use Argument when asking for user input (e.g. prompt) ?
Hello
I have done my program using the prompt method nested inside the function because I want to collect user input, but i saw the solution is using Argument to generate the random number. Is there a better way to do it? Anyway, do you guys have any feedback on the code I've written? Thanks!
function upperLower() {
var min = parseInt(prompt('Type the lowest possible value'));
var max = parseInt(prompt('Type the highest possible value'));
var random = Math.floor(Math.random() * (max - min + 1)) + min;
return random;
}
var randomNumber = upperLower();
alert('Your number is ' + randomNumber);
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsIf you wanted to use both, you would need to prompt the user for the two numbers outside of the function, and have the function only do the task of generating the random number. For example:
function generateRandomNumber(min, max) {
var random = Math.floor(Math.random() * (max - min + 1)) + min;
return random;
}
var min = parseInt(prompt('Type the lowest possible value'));
var max = parseInt(prompt('Type the highest possible value'));
var randomNumber = generateRandomNumber(min, max);
alert('Your number is ' + randomNumber);
Note that the variables min
and max
outside of the function are different to the parameters of the same name within the function.
You'd be better off in this case giving them different names, so as to avoid confusion:
function generateRandomNumber(min, max) {
var random = Math.floor(Math.random() * (max - min + 1)) + min;
return random;
}
var lowerLimit = parseInt(prompt('Type the lowest possible value'));
var upperLimit = parseInt(prompt('Type the highest possible value'));
var randomNumber = generateRandomNumber(lowerLimit , upperLimit);
alert('Your number is ' + randomNumber);
Naming things to be clear, concise and logical is one of the greatest challenges facing programmers everywhere! :)