Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial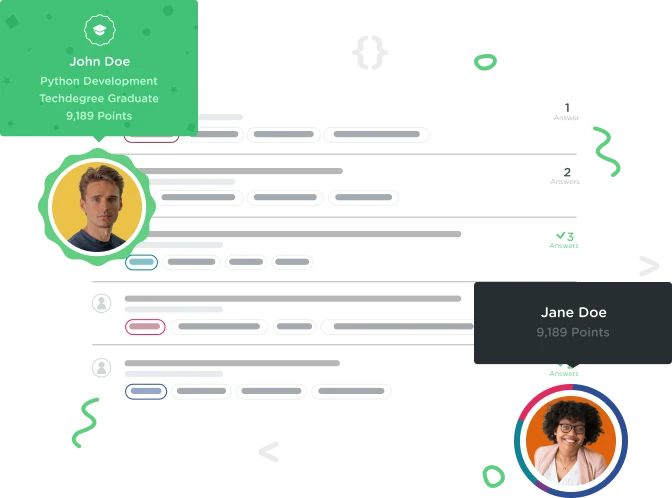

Martin Dweh
1,014 PointsHow can I use HEX COLOR CODES in Objective-C?
Hello Guys
I need a little help with add hex color in Objective-C
I have a simply app with a "colorsArray" set up, when I tap on a the button, the background color changes to the colors in the "colorsArray". The app works fine with the few colors names Xcode has.
But my problem here is; I want more colors - specific colors which names are not in Xcode.
How can I add more colors to my "colorArray" using Hex Color Code?
This is my colorsArray colorsArray = [[NSArray alloc] initWithObjects:[UIColor redColor], [UIColor purpleColor], [UIColor brownColor], [UIColor grayColor], nil];
I want to add these colors: #d8b9e5 , #ffc3a0 , #00d2f7, and more to my "colorArray"
I'm using Xcode 7 - Objective-C
Please help, I'll really appreciate it, -THANK YOU
3 Answers

Robert Baghai
5,492 PointsThere's some alternatives here.
One thing you can do if you really wanted to, was have an image which is just merely a .png image of the color you want. So if the picture is just say a square that is the color Gold... You can set the background image to that image, which will change the color of the, in this case button, background to Gold.
You can create your own color with the following code :
UIColor *myColor = [UIColor colorWithRed: 127 green:127 blue:127 alpha:1];
(mess around with your numbers to get to the color you want)
- If you specifically want hex values you can do is use the following method:
-
(NSString *)hexStringFromColor:(UIColor *)color { const CGFloat *components = CGColorGetComponents(color.CGColor); CGFloat r = components[0]; CGFloat g = components[1]; CGFloat b = components[2];
return [NSString stringWithFormat:@"#%02lX%02lX%02lX", lroundf(r * 255), lroundf(g * 255), lroundf(b * 255)]; }
Then.. do Step 2 and create your own color and call the method on that new color created. Ex)
UIColor *myColor = [UIColor colorWithRed: 127 green:127 blue:127 alpha:1]; [self hexStringFromColor: myColor];
Thats it. You can, NSLog(@"%@",[self hexStringFromColor: myColor]); to see the hex value with is 100% accurate. Hope this helps

Robert Baghai
5,492 PointsSorry.. step numbers got a little messed up when i posted this.

Robert Baghai
5,492 PointsSorry that was my first forum post here so not quite sure how to format things 100% the way I'm intending to yet. Okay i'll try to make this simpler. You currently have an array of UIColors that you wish to add more custom-like UIColors to.
/*create your custom color, vary the numbers to create the color you have in mind. Keep alpha at 1, it means it is fully visible (the opacity). You can google: UIColor with Red green blue alpha to get a better understanding of making your own color
*/
UIColor *myCustomColor = [UIColor colorWithRed:127 green:127 blue:127 alpha:1];
//now add that color to your array of UIColors
NSArray *arrayOfColors = [NSArray arrayWithObjects: [UIColor redColor], [UIColor blueColor], [UIColor purpleColor], myCustomColor, nil];
/*
use the arrayOfColors as you wish which contains the standard UIColors you have access to in addition to your newly created custom color
*/
Hope this is a littler easier.
Martin Dweh
1,014 PointsMartin Dweh
1,014 PointsHey Robert, hank you very much for taking the time to help me. I'll do appreciate it.
-But this is a little too confusing, I don't know what goes where with the above code. please do clean things up for me. Thanks
I simply want to know how to create an Array of HEX COLORS. and I want to use the HEX ARRAY COLORS as my background colors. Each time I press the button, the background color changes to one of the hex colors in the HEX COLORS ARRAY