Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial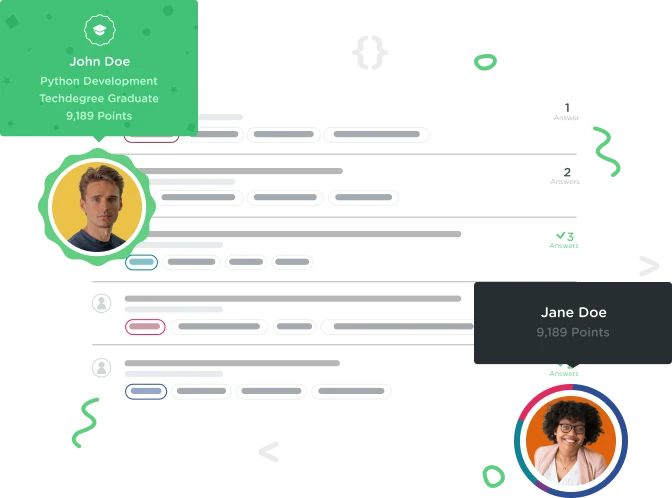

Leticia Snyder
619 PointsHow can I use loops to iterate and add to array?
numbers = []
number = 0
# write your loop here
loop do current_value(number)
numbers << number.add(1)
if numbers<3
break
end
2 Answers
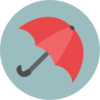
Nathan Brown
229 PointsHi Leticia,
I think that you are running into a problem because you are trying to do a little too much. You have almost everything you need, but also some additional things we won't need. We can simplify your solution.
loop do
is a great start. loop
is an iterator built into Ruby that will call its block forever( or until we tell it to break out of the loop). But it appears that you are also trying to write a method with current_value(number)
...that's one of the parts we can get rid of.
Inside of your do
block you definitely want to use the shovel operator << like you're doing to push the number into the array. But you don't want to use the dot operator .
to send the message add(1)
to number
. Instead you can add 1 to the number
variable and then reassign it to the variable number
which is a really complicated way of saying:
number = number + 1
Then one last thing. The code challenge wants you to break out of the loop before the array has more than three items. The way you've written it you are asking it to break out of the loop if the number is less than (<) 3. Since the number begins at zero it will break right away. We can have the loop break when it reaches 3 by writing
if number == 3
break
end
end
Notice that you will need two end
keywords, one to end the if statement and one to close the loop
block.
I will write the whole thing out for you so you can think through it and see the differences:
loop do
numbers << number
number = number + 1
if number == 3
break
end
end
Keep up the good work and let me know if you have any questions.
Nathan

Leticia Snyder
619 Pointsthank you so much.. I'm burnt out now.. but I will go through the code and your comments in the morning, I'm also studying full stack at Bloc, and I'm cross-pollinating for sure!!
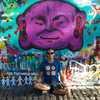
Andrew Stelmach
12,583 PointsKeep going Leticia! There's no clear path, but it DOES get easier! I've had some dark moments the last few months, but you WILL keep improving and become competent.