Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial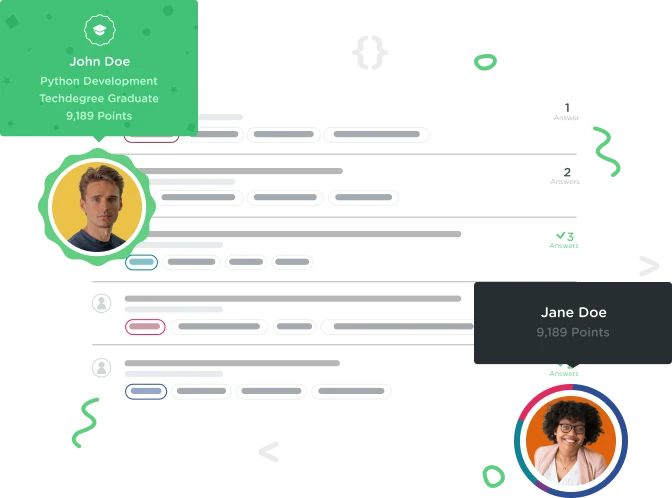

Federico Ades
934 PointsHow can I verify if the first letter of a String is equal to the letter 'm'?
I eeew to verify if the first letter in a String is the letter 'm'. If it isn't, I need todo the "throw new IllegalArgumentException" to inform the user that he must start the String with an m. This is the code I made: char m_first_name = fieldName.charAt(0); if (m_first_name != 'm') { throw new IllegalArgumentException ("A letter m in Lower Case is required in the first place of the world"); }
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
char m_first_name = fieldName.charAt(0);
if (m_first_name != 'm') {
throw new IllegalArgumentException ("A letter m in Lower Case is required in the first place of the world");
}
if (!Character.isLowerCase(fieldName.charAt(1))) {
Character.toUpperCase(fieldName.charAt(1));
}
return fieldName;
}
}
2 Answers
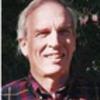
jcorum
71,830 PointsYou can use charAt(0) to get the first char in firstName, and Character.isUpperCase to check if the second character is upper case:
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (fieldName.charAt(0) != 'm' || !Character.isUpperCase(fieldName.charAt(1))) {
throw new IllegalArgumentException();
} else {
return fieldName;
}
}
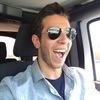
Anthony Mikhail
9,942 PointsI believe your method should work just fine, except you aren't looping to get a proper value out. Your method tells the user their input is wrong but doesn't ask them to input a new value. You could do something like:
while (fieldName.toLowerCase().indexOf("m") != 0){ //ask the user to input a new fieldName } return fieldName;

Federico Ades
934 PointsThank you very much!!
Federico Ades
934 PointsFederico Ades
934 PointsThank you very much!!