Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial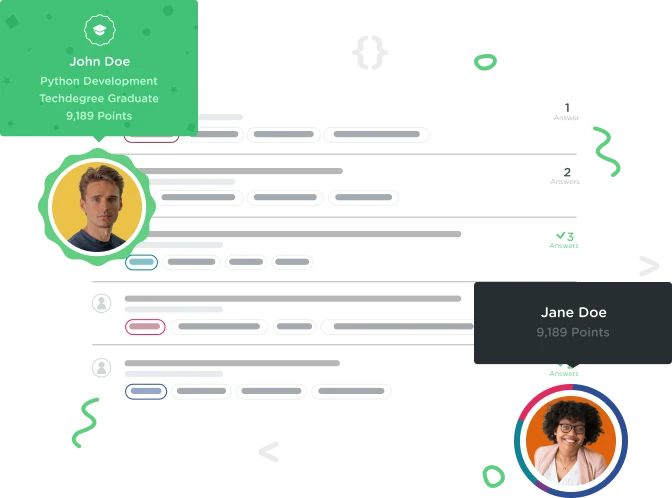

Jordan Wood
2,637 PointsHow can I wrap my text to span over multiple lines in order to fit within div?
I want to create a new version of my website to work on smaller screens such as Mobiles. The current version uses CSS animation to act as the text is being typed onto the screen. This is done line by line as you can see from the HTML classes "line1" "line2" etc.
This is ok with larger screens however when the screen size is smaller, the width of these lines is changing from device to device. I need to create a version where the text is wrapped within the "fakeScreen" container and can span across multiple lines.
Rather than having to define each line and its width text would be typed as paragraphs.
<head>
<link rel="shortcut icon" href="code.png" type="image/x-icon" />
<link rel="stylesheet" href="main.css">
<script type="text/javascript" src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
</head>
<div class="terminal">
<div class=fakeMenu>
<div class="fakeButtons fakeClose"></div>
<div class="fakeButtons fakeMinimize"></div>
<div class="fakeButtons fakeZoom"></div>
</div>
<div class="fakeScreen">
<p id="info-line"> </p>
<div class="css-typing">
<p class="typing line1">
text text text text text
</p>
<p class="typing line1">
text text text text text text text text text
</p>
<p class="typing line2>
text text text text text,
</p>
<p class="typing line4">
[?] What more would you like to know? Enter 0 for Skills, 1 for
</p>
<p class="typing line5">
Qualifications, 2 for About, 3 for Projects and 4 for Contact details.
</p>
</div>
</div>
</div>
</div>
body {
background: url("stars.jpg") no-repeat center center fixed;
-webkit-background-size: cover;
-moz-background-size: cover;
-o-background-size: cover;
background-size: cover;
padding: 10px;
}
.terminal{
margin-top: 5%;
}
.fakeButtons {
height: 10px;
width: 10px;
border-radius: 50%;
border: 1px solid #000;
position: relative;
top: 6px;
left: 6px;
background-color: #ff3b47;
border-color: #9d252b;
display: inline-block;
}
.fakeMinimize {
left: 11px;
background-color: #ffc100;
border-color: #9d802c;
}
.fakeZoom {
left: 16px;
background-color: #00d742;
border-color: #049931;
}
.fakeMenu {
width: 728px;
box-sizing: border-box;
height: 25px;
background-color: #bbb;
margin: 0 auto;
border-top-right-radius: 5px;
border-top-left-radius: 5px;
}
.fakeScreen {
background-color: #151515;
box-sizing: border-box;
width: 728px;
height: 457px;
margin: 0 auto;
padding: 1px 0 0 11px;
border-bottom-left-radius: 5px;
border-bottom-right-radius: 5px;
overflow: -moz-scrollbars-vertical;
overflow-y: auto;
overflow-x: hidden;
}
span{
color: white;
font-family: monospace;
font-size: 1.25em;
font-weight: bold;
}
#info-line{
position: relative;
text-align: left;
font-size: 1.25em;
font-family: monospace;
white-space: normal;
overflow: hidden;
color: white;
}
.css-typing p.typing {
border-right: .15em solid white;
font-family: monospace;
font-size: 1.25em;
white-space: nowrap;
overflow: hidden;
}
.css-typing p.line1 {
width: 250px;
-webkit-animation: type 2s steps(40, end); /* Type of animation and steps of animation tranistion */
animation: type 2s steps(40, end);
-webkit-animation-fill-mode: forwards;
animation-fill-mode: forwards;
color: white;
}
.css-typing p.line2 {
width: auto;
opacity: 0;
-webkit-animation: type2 3s steps(40, end);
animation: type2 3s steps(40, end);
-webkit-animation-delay: 2s;
animation-delay: 2s;
-webkit-animation-fill-mode: forwards;
animation-fill-mode: forwards;
color: #CDEE69;
}
.css-typing p.line3 {
width: 371px;
opacity: 0;
-webkit-animation: type2 3s steps(40, end);
animation: type2 3s steps(40, end);
-webkit-animation-delay: 5s;
animation-delay: 5s;
-webkit-animation-fill-mode: forwards;
animation-fill-mode: forwards;
color: #CDEE69;
margin-top: -16px;
}
.css-typing p.line4 {
width: 623px;
opacity: 0;
-webkit-animation: type2 1s steps(1, end);
animation: type2 1s steps(1, end);
-webkit-animation-delay: 8s;
animation-delay: 8s;
-webkit-animation-fill-mode: forwards;
animation-fill-mode: forwards;
color: #E09690;
border: 0 !important;
}
.css-typing p.line5 {
width: 686px;
opacity: 0;
-webkit-animation: type3 1s steps(1, end), blink .5s step-end infinite alternate;
animation: type3 1s steps(1, end), blink .5s step-end infinite alternate;
-webkit-animation-delay: 8s;
animation-delay: 8s;
-webkit-animation-fill-mode: forwards;
animation-fill-mode: forwards;
color: #E09690;
border: 0 !important;
margin-top: -16px;
}
.prompt{
font-size: 1.25em;
font-family: monospace;
color: white;
width: 675px;
background-color: #151515;
border: 0;
outline: none;
}
.result{
font-size: 1.25em;
font-family: monospace;
color: #9CD9F0;
width: 675px;
}
.not-found{
color: #E09690;
}
/* Typing animation */
@keyframes type {
0% {
width: 0;
}
99.9% {
border-right: .15em solid white;
}
100% {
border: none;
}
}
@-webkit-keyframes type {
0% {
width: 0;
}
99.9% {
border-right: .15em solid white;
}
100% {
border: none;
}
}
@keyframes type2 {
0% {
width: 0;
}
1% {
opacity: 1;
}
99.9% {
border-right: .15em solid white;
}
100% {
opacity: 1;
border: none;
}
}
@-webkit-keyframes type2 {
0% {
width: 0;
}
1% {
opacity: 1;
}
99.9% {
border-right: .15em solid white;
}
100% {
opacity: 1;
border: none;
}
}
@keyframes type3 {
0% {
width: 0;
}
1% {
opacity: 1;
}
100% {
opacity: 1;
border: none;
}
}
@-webkit-keyframes type3 {
0% {
width: 0;
}
1% {
opacity: 1;
}
100% {
opacity: 1;
border: none;
}
}
/* prompt cursor blink */
@keyframes blink-animation {
to {
visibility: hidden;
}
}
@-webkit-keyframes blink-animation {
50% {
visibility: hidden;
}
}
/* Media Query CSS */
/* Mobile */
@media only screen and (max-width: 400px){
body{
margin: 0;
padding: 0;
}
.terminal{
padding: 15px;
margin: 0;
}
.fakeMenu{
width: 100%;
}
.fakeScreen{
width: 100%;
height: 100%;
}
.prompt{
width: auto;
}
#info-line{
font-size: 1em;
}
}
1 Answer
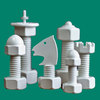
Steven Parker
230,274 PointsText wrapping is a normal aspect of "flow" content in an HTML document. You're not seeing it in this example because you've specifically separated lines into separate elements.
More typically, a paragraph would be a single element, and wrapping would happen automatically:
<p class="result">
[?] What more would you like to know? Enter 0 for Skills,
1 for Qualifications,2 for About, 3 for Projects and 4 for Contact details.
</p>
Of course, this will look pretty strange if you apply one of the animation effects intended for block elements. So if the animation is important you may need to find another way to achieve it (perhaps using JavaScript).