Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial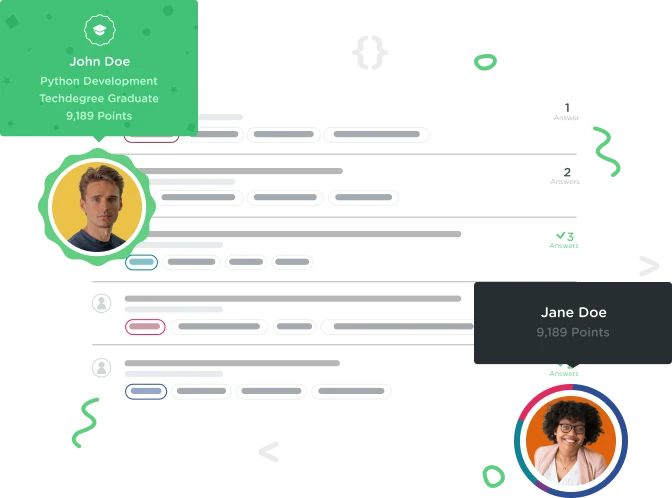

Lana Wong
3,968 PointsHow can I write my code with a failable initializer?
HI. Pasan said to practice once we finished the enumerations and optionals course.
I wrote this code:
struct superHero{
let name: String
let power: String
let city: String?
func new() -> superHero?{
if name != nil, power != nil, city != nil{
return superHero(name: name, power: power, city: city)
}else{
return nil
}
}
}
//Instances
let someHero = superHero(name: "Spider Man", power: "Shoots spider webs", city: "New York")
let anotherHero = superHero(name: "Wonder Woman", power: "Amazon fighting skills", city: nil)
First of all, how can I write this code with a failable initializer? Second, is this object considered "safe"?
1 Answer
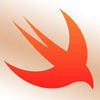
Jeff McDivitt
23,970 PointsIn your code since name and power are not optional they must have a value, you will probably get a warning in Xcode saying that the value will always be true.
Here is how you write it with a failable initializer
struct superHero{
let name: String
let power: String
let city: String?
init?(name: String, power: String, city: String) {
if city.isEmpty { return nil }
self.name = name
self.power = power
self.city = city
}
}
let someHero = superHero(name: "Spider Man", power: "Shoots spider webs", city: "New York")
let anotherHero = superHero(name: "Wonder Woman", power: "Amazon fighting skills", city: "")
Lana Wong
3,968 PointsLana Wong
3,968 PointsWhat is the city.isEmpty?