Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial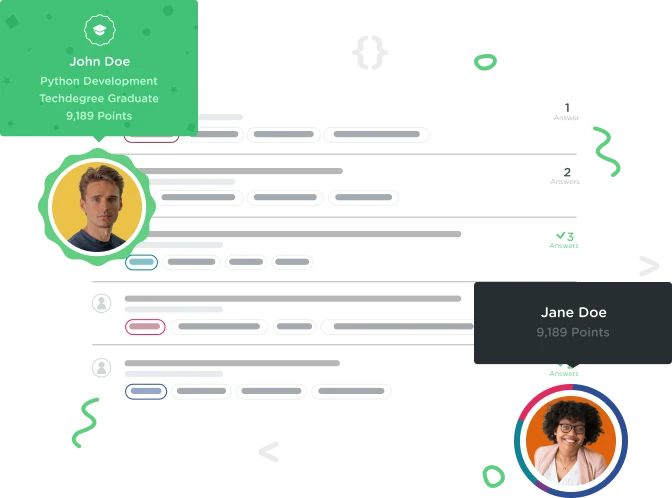

Henry Stiltner
4,178 PointsHow can my code be improved?
How can I do better?
"use strict";
let correctAnswers = 0;
let wrongAnswers = 0;
let correctQuestions = [];
let wrongQuestions = [];
const questions =
[
['What color is the sky?', 'blue'],
['What color is grass?', 'green'],
['What color are clouds?', 'white']
];
function printQuestions(list)
{
let listHTML = "<ol>";
for (let i = 0; i < list.length; i++)
{
listHTML += `<li>${list[i]}</li>`;
}
listHTML += "</ol>";
print(listHTML);
}
function print(message)
{
let outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
for (let i = 0; i < questions.length; i++)
{
let answer = prompt(questions[i][0]);
if (answer === questions[i][1])
{
correctQuestions.push(questions[i][0]);
correctAnswers++;
}
else
{
wrongQuestions.push(questions[i][0]);
wrongAnswers++;
}
}
print(`<h2>You got ${correctAnswers} right and ${wrongAnswers} wrong.</h2>`);
print(`<h2>You got these questions right.</h2>`);
printQuestions(correctQuestions);
print(`<h2>You got these questions wrong.</h2>`);
printQuestions(wrongQuestions);
1 Answer
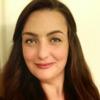
Jennifer Nordell
Treehouse TeacherHi there! Your code is actually really good. However, if I were going to be really picky, I'd point out that you have two variables which actually aren't needed at all. But this is strictly my opinion.
You have two variables which hold an integer representing the number correct and the number incorrect. You have two arrays which will store the correctQuestions
and wrongQuestions
. Arrays have a built in property called length, whcih will ultimately hold the same numbers you're using in your correctAnswers
and wrongAnswers
. So I took the liberty of reworking your code a bit with my suggestion.
"use strict";
// let correctAnswers = 0;
// let wrongAnswers = 0;
let correctQuestions = [];
let wrongQuestions = [];
const questions =
[
['What color is the sky?', 'blue'],
['What color is grass?', 'green'],
['What color are clouds?', 'white']
];
function printQuestions(list)
{
let listHTML = "<ol>";
for (let i = 0; i < list.length; i++)
{
listHTML += `<li>${list[i]}</li>`;
}
listHTML += "</ol>";
print(listHTML);
}
function print(message)
{
let outputDiv = document.getElementById('output');
outputDiv.innerHTML += message;
}
for (let i = 0; i < questions.length; i++)
{
let answer = prompt(questions[i][0]);
if (answer === questions[i][1])
{
correctQuestions.push(questions[i][0]);
// correctAnswers++; no longer needed
}
else
{
wrongQuestions.push(questions[i][0]);
// wrongAnswers++; no longer needed
}
}
// access the length properties on the arrays to get the number correct and incorrect
print(`<h2>You got ${correctQuestions.length} right and ${wrongQuestions.length} wrong.</h2>`);
print(`<h2>You got these questions right.</h2>`);
printQuestions(correctQuestions);
print(`<h2>You got these questions wrong.</h2>`);
printQuestions(wrongQuestions);
Hope this helps (and makes sense)!
Henry Stiltner
4,178 PointsHenry Stiltner
4,178 PointsVery clever, Thanks!