Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial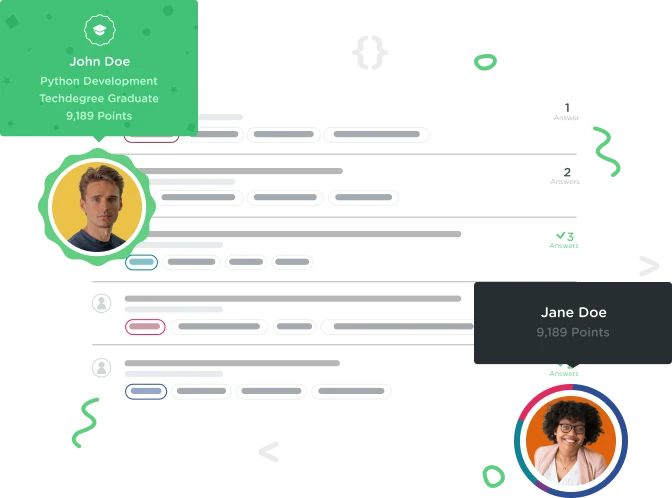
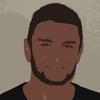
jsdevtom
16,963 PointsHow can non numbers be removed from lists in Python?
Please note, I do not mean 'check if a string object is a digit' e.g '8'
.
The list:
my_list = [5, 2, 1, True, 'abcdefg', 3, False, 4]
What I've tried so far:
# Returns 'AttributeError: 'int' object has no attribute 'isDigit''
my_list = [x for x in my_list if x.isDigit()]
# Returns 'ValueError: invalid literal for int() with base 10: 'abcdefg''
my_list = [x for x in my_list if int(x)]
I know that in Javascript, the following code would achieve what I want to do:
var my_list = [5, 2, 1, true, 'abcdefg', 3, false, 4]
var filtered = my_list.filter(function(item) {
return !isNaN(parseFloat(item)) && isFinite(item)
})
console.log(filtered);
Thanks in advance
2 Answers

Idan Melamed
16,285 PointsHere's how you would do it:
my_list = [5, 2, 1, True, 'abcdefg', 3, False, 4]
my_list = [x for x in my_list if type(x) == int]

Sahil Sharma
4,791 Pointsisdigit() function returns True or False if there are only numbers in ---> String not for integers themselves, as the elements of my_list contain integers too, so isdigit() returns an error, you can fix that by if str(x).isdigit().

Sahil Sharma
4,791 PointsOh, and I think you cannot use isDigit(), instead use isdigit() function calls are case-sensitive.
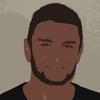
jsdevtom
16,963 PointsThank you :-)
jsdevtom
16,963 Pointsjsdevtom
16,963 PointsThanks. I added all numeric tpes to account for all types of numbers as so: