Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial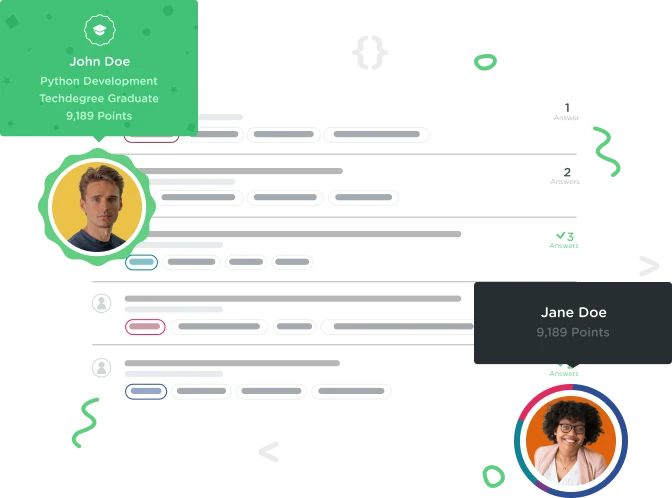

iworld
4,125 PointsHow can we do additional testing for python internal like __repr__ ?
I would like to know how we can tests internals like __repr__
.
Are there any examples?
Testing:
def __repr__(self):
return str(self.value)
I tried assertIsInstance
but the report says it is not working for __repr__
.
2 Answers

Jon Mirow
9,864 PointsHi there!
Short story:
you can just use the built in function repr() to get the value returned by the __repr
__ method. Note that repr()'s job is really just to represent the instance as a string, but as the name suggests, if a class has a __repr
__ method, it will return what's in there, for example:
>>>test = SomeClass()
>>>print(repr(test))
<SomeClass object at 0x7f57e1444390>
Long story:
If it's okay with you, I'll handle __repr
__ and __str
__ (from now on I'll just call them repr and str just because it's too annoying to keep escaping them from the markdown lol) together. Both are kind of similar, and actually have the same default value for a user defined class - they just return self. That value is:
<x object at y>
# where
# x = the class name
# y = the memory address the instance is stored at
We can test that here:
class Test:
value = "Test Data"
instance = Test()
print(instance)
print(repr(instance))
Will print something like:
<Test object at 0x7f57e1418438>
<Test object at 0x7f57e1418438>
People often get confused about the difference between str and repr (I've heard it's often an interview test!) . Bascially repr is the formal/official/internal name to give an instance, and str is the informal/readable/end user name to display to users. For example, python's built in types - int, float, str, dict, etc,etc return the same information from repr and str, because that's the most important information - not where the int with the value 72 is in memory, but that it's the value 72. However for our own defined classes, like Test above, we often want a publicly facing name, that gives the user the important information, and an internal name that gives the developer a bit more info.
class Test:
value = "Test Data"
def __str__(self):
return self.value
def __repr__(self):
return "<Instance of {} class, value={}>".format(self.__class__.__name__, self.value)
instance = Test()
print(instance)
print(repr(instance))
Will print:
Test Data
<Instance of Test class, value=Test Data>
Of course, they can print anything, it's just an example... all that matters is to remember repr == internal representation, str == external
Hope it helps :)

Paema Hare
22,706 PointsHi! to test that code with unittest:
def test_die_repr(self):
repr(self.d8)