Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial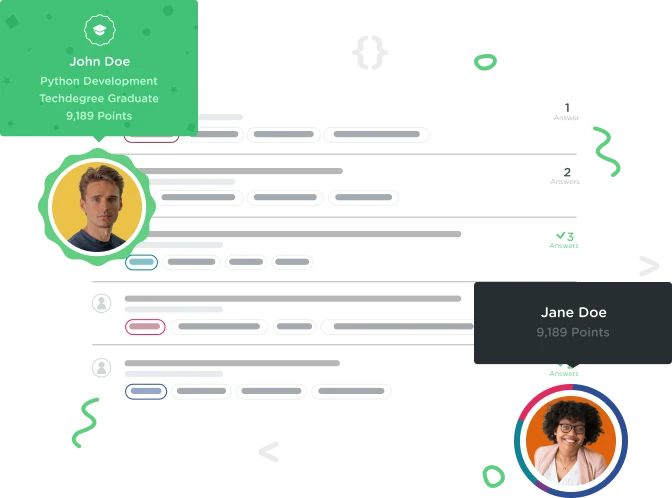
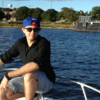
Evan N
5,097 PointsHow can we prevent this program from running as a loop?
I used some different variable names but otherwise followed along with the videos on my local machine. The issue, is that it's running as a loop until (I assume) that there's 0 tickets left. How can I modify this or is this how the program is supposed to run?
Notes: My environment is currently running python 3.6.0
tickets_remaining = 100
SERVICE_CHARGE = 2
TICKET_PRICE = 10
def calculate_price(number_of_tickets):
return (number_of_tickets * TICKET_PRICE) + SERVICE_CHARGE
while tickets_remaining >= 1:
print("There are {} tickets remaining".format(tickets_remaining))
name = input("What is your name? ")
tickets_purchased = int(input("Hey {}, how many tickets do you want to buy? ".format(name)))
try:
tickets_purchased = int(tickets_purchased)
if tickets_purchased > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
except ValueError as err:
print("Oh no, we ran into an issue {} please try again.".format(err))
else:
tickets_purchased_price = calculate_price(tickets_purchased)
print("You owe ${}".format(tickets_purchased_price))
should_proceed = input("Do you want to proceed with this purchase? Y/N ")
if should_proceed.lower() == "y":
print("SOLD!")
tickets_remaining -= tickets_purchased
else:
print("Thank you anyways, {}!".format(name))
print("end of program")
2 Answers
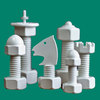
Steven Parker
230,274 PointsIf you only want it to run one time, you could remove the "while" line and shift the indentation of everything else to the left.
Or if you want it to loop until you type "stop" instead of a name, you could add a test after the name is input and compare it to "stop". Then if it matches you could perform a "break" to end the loop.
These are just two options, how do you want the program to perform?

issam king
Courses Plus Student 744 Pointsthe problem is if you choose the "break" option , once the test is made it throws you to the print(sold out) while the tickets could still be there
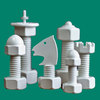
Steven Parker
230,274 PointsYou must be looking at different code. A "break" in the code above would take you directly to the "end of program" message.
Cooper Runstein
11,850 PointsCooper Runstein
11,850 PointsIf you format your question as code we'll have a much easier time understanding whats going on. Use three ` marks above your code to format it as such.