Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial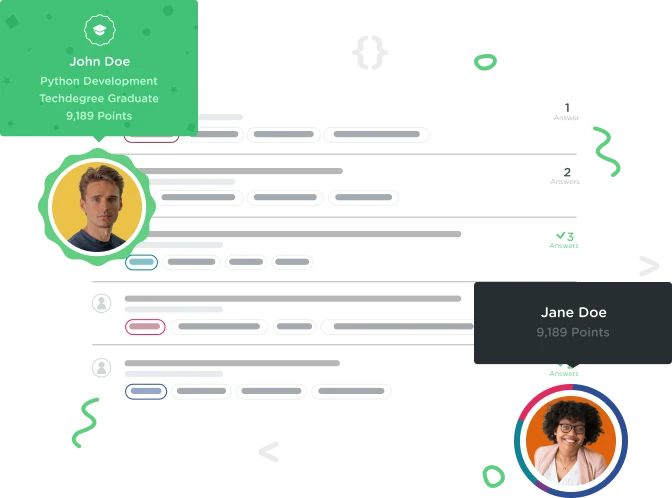

Gavin Murphy
10,004 PointsHow can you specifically target an array for comparison? I can rule out undefined and Not a Num but can't rule out str
I am a little stuck here. would be grateful for a hand. cheers
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter(array) {
if(array === undefined || array === NaN || array === "aString") {
return 0;
}
return array.length;
}
</script>
</body>
</html>
1 Answer

Kevin Lozandier
Courses Plus Student 53,747 PointsHi, Gavin Murphy:
Objects and Arrays have traditionally have had quirky behavior in JavaScript before ES6. For example, the following will output "What is going on?"
regardless of comparison check strictness [==
w/ (type coercion) or ===
(with no type coercion)]
var arrayOfNumbers = [0, 1, 2, 3];
if ( arrayOfNumbers === [0, 1, 2, 3] ){
console.log("equal arrays");
} else {
console.log("What is going on?");
}
For that reason, many JS developers have relied on custom deepEqual
or isEqual
helper functions from libraries such as underscore that essentially check if two things are equal, considering if they're primitive type values (number, string, boolean, null, undefined, symbols) or reference type values (Objects such as functions, and so on).
As far as arrays, if the first object is an array, such functions will check if the second parameter is also an array and of the same length. From there, each item in an array in the first array is compared to the value with the same index if the first test(s) pass.
If both values are reference types, both are iterated again till equality can be truly verified, returning false immediately if that can be verified.
For what it's worth, including the quirkiness that is associated with NaN
as well, the committee that creates the standards for EcmaScript6 (something that is then adhered to by the next version of JavaScript) has specified plans for a Object.is
method being available to JavaScript developers that should minimize the need for third-party libraries or custom methods from scratch dealing with this
However, some transpilers or some browsers with Object.is
support may still not produce a reliable result.
For example I got the wrong output again when I used the implementation available on ES6Fiddle.net, a popular resource to try out ECMAScript6 JavaScript (ES6 JavaScript) concepts:
let arrayOfNumbers = [0, 1, 2, 3];
if ( Object.is( arrayOfNumbers, [0, 1, 2, 3]) ){
console.log("equal arrays");
} else {
console.log("What is going on?");
}
Accordingly, I recommend taking advantage of the helper methods that address this problem from lodash
or underscore
in the meantime.
For more information on this subject, I recommend this StackOverflow post