Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial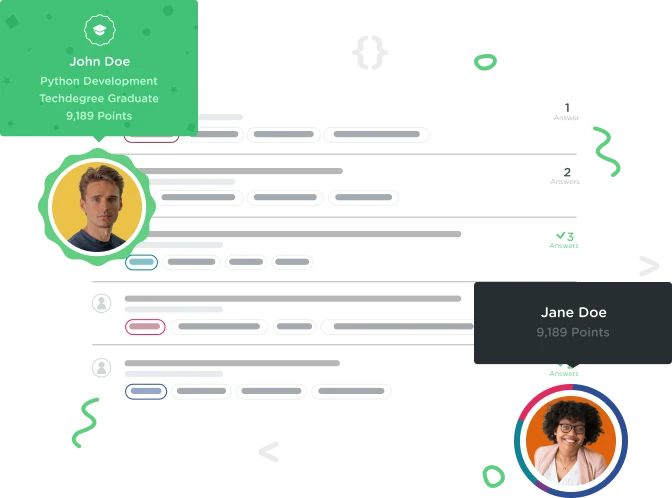

tian jin
Courses Plus Student 5,162 Pointshow checkbox event bind to checkBoxEventHandler?
I mean I know this line: " checkBox.onchange = checkBoxEventHandler; " bind the check box event, but how? You bind other elements with explicit method, like "editButton.onclick=editTask", but checkBoxEventHandler is just a parameter, how does the program know which method checkbox is calling? Why it knows it's calling either taskCompleted or taskIncomplete?? I hope the question is clear.
//Problem: User interaction doesn't provide desired results.
//Solution: Add interactivty so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTaskHolder = document.getElementById("incomplete-tasks");//incomplete-tasks
var completedTaskHolder = document.getElementById("completed-tasks");//completed-tasks
//functions*****************************************************************************//
//new task list item
var createNewTaskElement = function(taskString){
//cereate list item
var listItem = document.createElement("li");
//input checkbox
var checkBox = document.createElement("input");
//label
var label = document.createElement("label");
//input text
var editInput = document.createElement("input");
//button edit
var editButton = document.createElement("button");
//button delete
var deleteButton = document.createElement("button");
//each element needs be modifing
//each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//add a new task
var addTask = function(){
var listItem = createNewTaskElement("Some new task");
//append listItem to incompleteTaskHolder
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem,taskCompleted);
}
//edit an exsisiting task
var editTask = function(){
console.log("e");
}
//delete an existing task
var deleteTask = function(){
console.log("d");
}
//mark a task as complete
var taskCompleted = function(){
var listItem = this.parentNode;
completedTaskHolder.appendChild(listItem);
bindTaskEvents(listItem,taskIncomplete);
}
//mark a task as incomplete
var taskIncomplete = function(){
//append the task list item to the #completed-task
var listItem = this.parentNode;
incompleteTaskHolder.appendChild(listItem);
bindTaskEvents(listItem,taskCompleted);
}
var bindTaskEvents = function (taskListItem,checkBoxEventHandler){
console.log("bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
editButton.onclick = editTask;
deleteButton.onclick = deleteTask;
checkBox.onchange = checkBoxEventHandler;
}
//set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for(var i =0; i < incompleteTaskHolder.children.length; i++){
//bind events to list item's children(taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i],taskCompleted);
}
//cycle over completeTasksHolder ul list items
for(var i =0; i < completedTaskHolder.children.length; i++){
//bind events to list item's children(taskCompleted)
bindTaskEvents(completedTaskHolder.children[i],taskIncomplete);
}
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi tian jin ,
You want to look at where the bindTaskEvents
function is being called in order to figure out what is being passed in for that second argument.
It's being called in the 2 for loops at the bottom of the code:
//cycle over incompleteTasksHolder ul list items
for(var i =0; i < incompleteTaskHolder.children.length; i++){
//bind events to list item's children(taskCompleted)
bindTaskEvents(incompleteTaskHolder.children[i],taskCompleted);
}
//cycle over completeTasksHolder ul list items
for(var i =0; i < completedTaskHolder.children.length; i++){
//bind events to list item's children(taskCompleted)
bindTaskEvents(completedTaskHolder.children[i],taskIncomplete);
}
In the first for loop the bindTaskEvents
function is being called with the taskCompleted
function as the second argument. This means that the taskCompleted
function will be assigned to the change event for the checkboxes in those particular list items.
The same thing happens in the second loop except with the taskIncomplete
function instead.

tian jin
Courses Plus Student 5,162 Pointsthanks Jason!

Stephanos Philippides
11,521 Pointsvar bindTaskEvents = function (taskListItem,checkBoxEventHandler){
console.log("bind list item events");
checkBox.onchange = checkBoxEventHandler;
}
An example of how you would use this is if you wanted to make the check box alert something you would have
var someFunction = function(){
alert("I am a function");
}
then you would pass someFunction as a parameter for the bind function like this
bindTaskEvents(parameterForListItem, someFunction);
so when you change the checkbox it would trigger the event handler wich in this case is someFunction so it would alert "I am a function".
Hope this helped
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHey tian jin,
I edited your code so that it will render properly on the forum. You can read the Markdown Cheatsheet to post code and see the following image for how to post code: