Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial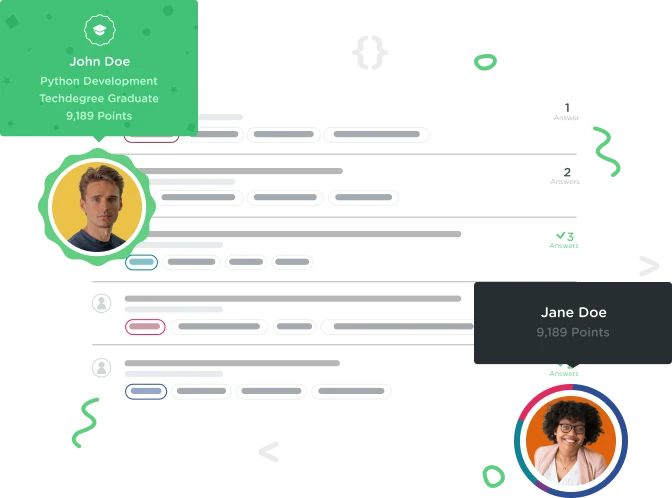

Craig Brah
Courses Plus Student 1,995 PointsHow come changing the indexes didn't change the order of the list items?
When the foreach function was used to iterate through the array, shouldn't it start at the lowest index in the array and then go on to the next one? So when the indexes were changed how come the order of the list items stayed the same even though the last list item has the second lowest index?
Here is the code for an example:
Creating the array and setting the indexes:
<?php
$catalog = array();
$catalog[101] = "Design Patterns";
$catalog[201] = "Forest Gump";
$catalog[301] = "Beethoven";
$catalog[102] = "Clean Code";
?>
Then iterating through the array to add it to an unordered list:
<?php
foreach($catalog as $item){
echo "<li>" . $item . "</li>";
}
?>
Shouldn't the outcome be:
<ul>
<li>Design Patterns</li>
<li>Clean Code</li>
<li>Forest Gump</li>
<li>Beethoven</li>
</ul>
But instead it comes out:
<ul>
<li>Design Patterns</li>
<li>Beethoven</li>
<li>Forest Gump</li>
<li>Clean Code</li>
</ul>
So why is this? Does foreach not start at the lowest index but instead goes through the order in which the items were added to the array? If so how does it know to do that and why doesn't it start at the lowest index?
Thanks.
1 Answer
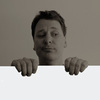
Sean T. Unwin
28,690 PointsThe parsing of an array is typically based upon the order which it was created, as you have seen from your example. If you want to sort an array by key then use ksort(). This would be placed after the array was created, but before the foreach function. Usage: ksort($catalog);
For further information on array sorting see the Comparison of Array Sorting Functions.
edit: Added a Snapshot to view an example; fork it to run.
Craig Brah
Courses Plus Student 1,995 PointsCraig Brah
Courses Plus Student 1,995 PointsI was not aware of ksort() so thank you very much for your answer and for the snapshot, cheers.