Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial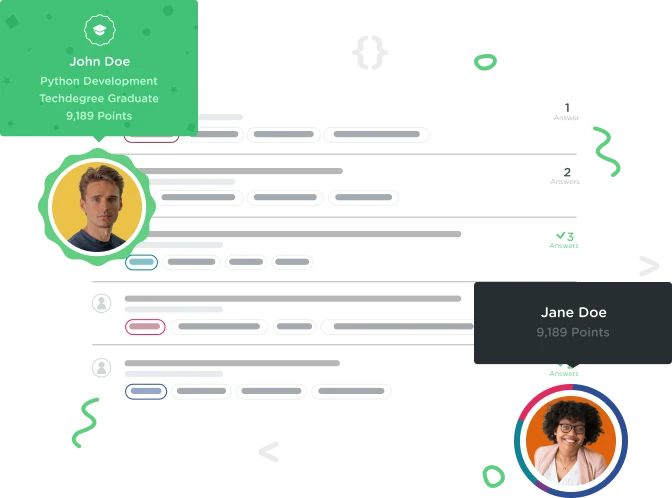

Fernando Jimenez
Courses Plus Student 8,455 PointsHow come is not returning p as the nextElementSibiling?
I can't get this to grab the p next to li so I can add the class "highlight"
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
list.nextElementSibling.addClass("highlight");
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button>Highlight</button></li>
<li><p>Events</p><button>Highlight</button></li>
<li><p>Event Listening</p><button>Highlight</button></li>
<li><p>DOM Traversal</p><button>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
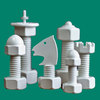
Steven Parker
231,269 PointsYou'll want to base your traversal on the click target, not the list itself.
But also, when I look at the HTML, those paragraphs seem to be previous to the buttons, not next after them.

Fernando Jimenez
Courses Plus Student 8,455 Pointswhat If i try this inside the if statement?
e.target.tagname.previousSibling.addClass(".highlight");
I tried but it didn't work, the console is not recognizing what target is.
Inside that if statement, does "target.tagName == ' <button>' ? o do I have to say e.target.tagname?
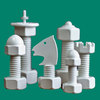
Steven Parker
231,269 PointsI added a comment to my answer.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsSo, if you currently have:
e.target.tagname.previousSibling.addClass(".highlight");
It looks like a few extra things happened during that change:
And yes, you need "e.target". Just "target" would be undefined.
Fernando Jimenez
Courses Plus Student 8,455 PointsFernando Jimenez
Courses Plus Student 8,455 PointsSo I tried
var list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function (e) { if (e.target.tagName == 'BUTTON') { e.target.tagname.previousElementSibling.classList.add("highlight"); } });
but still get it wrong, it seems that I am not using previousElementSibling properly.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsPerhaps you overlooked the first of those hints .. you still have "tagname" in your traversal.
Fernando Jimenez
Courses Plus Student 8,455 PointsFernando Jimenez
Courses Plus Student 8,455 Pointsvar list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function (e) { if (e.target.tagName == 'BUTTON') { e.target.previousElementSibling.classList.add("highlight"); } });
I am still getting an error even after getting rid of tagname; not sure whats going on to be honest.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsNow it looks right to me. So I tested it by copy/pasting your line directly into the challenge and it passed!
Maybe try a browser restart?
Fernando Jimenez
Courses Plus Student 8,455 PointsFernando Jimenez
Courses Plus Student 8,455 Pointswierd, it gave me an error on the console, but ok...
Thanks!
Steven Parker
231,269 PointsSteven Parker
231,269 PointsBut did it also pass the challenge?