Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial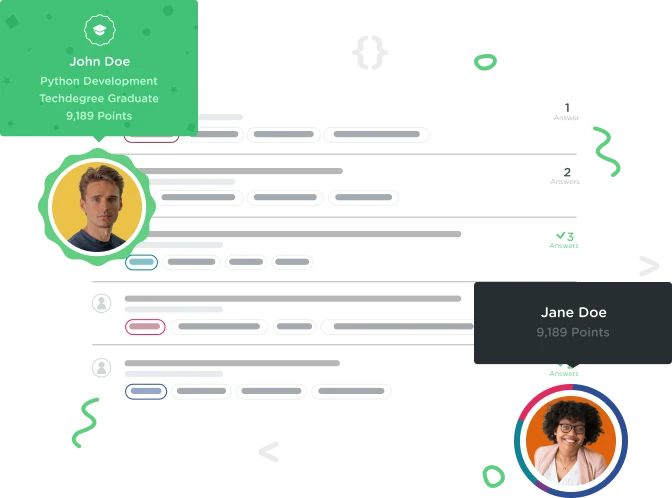

Mykiel Horn
4,014 PointsHow come my function isn't outputting the right number of prints?
I placed all of my code into workspaces and when I run my code it does what I think is correct. It prints 5 random numbers and tells me are they even or odd. But when I run my code in the Code Challenge, it says that my code has the wrong number of prints. Can anyone tell me what am I missing so I can complete this code challenge?
import random
start = 5
def even_odd(start):
while start > 0:
rannum = random.randint(1,99)
if rannum%2 == 0:
print("{} is even".format(rannum))
else:
print("{} is odd".format(rannum))
start = start - 1
return
2 Answers
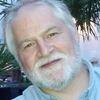
Jeff Muday
Treehouse Moderator 28,720 PointsYou got most of the code correct. But your interpretation of the challenge needs to be modified.
They are asking you to treat even_odd() as a stand-alone function and build your code to utilize the function is a checker for even and odd numbers.
import random
# leave even_odd just like they gave it to you.
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
# your challenge code goes here.
start = 5
while start:
rannum = random.randint(1,99)
if even_odd(rannum):
print("{} is even".format(rannum))
else:
print("{} is odd".format(rannum))
start = start - 1
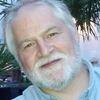
Jeff Muday
Treehouse Moderator 28,720 PointsYes, you can do it the alternate way of modifying even_odd, it will also pass the challenge. So, as with any challenge, there are all different ways to write them. In this case, Mykeil's modification of even_odd will pass muster.
see below:
import random
def even_odd(num):
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
while num:
rannum = random.randint(1,99)
if rannum % 2:
print("{} is odd".format(rannum))
else:
print("{} is even".format(rannum))
num -= 1
start=5
even_odd(start)
Rob Enderle
2,164 PointsRob Enderle
2,164 PointsI believe they wanted you to create the while loop outside and not modify the function, although your code does produce the intended result when called.
You'd need to put
even_odd(start)
outside, after your function.
If you wanted to use the function as provided, you could do something like the following: