Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial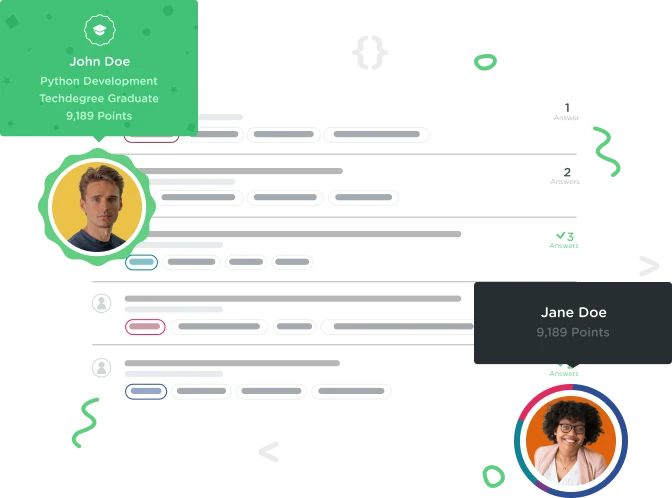

Edmund Yu
Full Stack JavaScript Techdegree Student 4,053 PointsHow come the correct/incorrect alert only comes up after all questions are asked?
Shouldn't the correct/incorrect alert prompt after I answer the question? Why isn't this so? Below is my code.
var questionOne = prompt("What is your favorite ice cream?"); var questionTwo = prompt("What is your favorite car?"); var questionThree = prompt("What is your favorite color?"); var questionFour = prompt("What is your favorite clothing brand?"); var questionFive = prompt("What is your favorite dog breed?");
var correct = 0; var questionTracker = 0;
var correctAns1 = "chocolate"; var correctAns2 = "AE86"; var correctAns3 = "red"; var correctAns4 = "BAPE"; var correctAns5 = "husky";
if (questionTracker === 0) { questionOne; if (questionOne.toLowerCase() == correctAns1) { alert("Correct!"); correct += 1;
}
else { alert("Incorrect! Please move on to the next question.");
} questionTracker += 1; }
if (questionTracker === 1) { questionTwo; if (questionTwo.toUpperCase() == correctAns2) { alert("Correct!"); correct += 1;
}
else { alert("Incorrect! Please move on to the next question.");
} questionTracker += 1; }
if (questionTracker === 2) { questionThree;
if (questionThree.toLowerCase() == correctAns3) { alert("Correct!"); correct += 1;
}
else { alert("Incorrect! Please move on to the next question."); } questionTracker += 1; }
if (questionTracker === 3) { questionFour; if (questionFour.toUpperCase() == correctAns4) { alert("Correct!"); correct += 1;
}
else { alert("Incorrect! Please move on to the next question.");
} questionTracker += 1; }
if (questionTracker === 4) { questionFive; if (questionFive.toLowerCase() == correctAns5) { alert("Correct!"); correct += 1;
}
else { alert("Incorrect! Please move on to the next question.");
} questionTracker += 1; }
if (correct === 5) { document.write("<p>Congratulations! You have gotten " + correct + " questions correct! </p>"); } else if (correct === 4) { document.write("<p>Congratulations! You have gotten " + correct + " questions correct! </p>"); } else if (correct === 3) { document.write("<p>Congratulations! You have gotten " + correct + " questions correct! </p>"); } else if (correct === 2) { document.write("<p>Congratulations! You have gotten " + correct + " questions correct! </p>"); } else if (correct === 1) { document.write("<p>Congratulations! You have gotten " + correct + " questions correct! </p>"); } else { document.write("<p> You suck! </p>"); }
3 Answers

KRIS NIKOLAISEN
54,971 PointsYour variable declarations (listed one after the other) is where the user is prompted.
var questionOne = prompt("What is your favorite ice cream?")
if (questionTracker === 0) {
questionOne; /*this line does nothing*/
if (questionOne.toLowerCase() == correctAns1) {
alert("Correct!"); correct += 1;
}
else { alert("Incorrect! Please move on to the next question.");
}
questionTracker += 1;
}
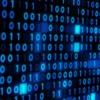
Alexander Davison
65,469 PointsAll of the if
statements are after the prompt
s, so no, it won't print the alert
s right after each prompt
.
Try something like this:
var questionOne = prompt(...);
if (questionOne == ...) {
alert("Correct!");
numberCorrect++;
} else {
alert("Incorrect! Please move on to the next question.");
}
...etc...

Edmund Yu
Full Stack JavaScript Techdegree Student 4,053 PointsOK! Thanks guys!
Ross King
20,704 PointsRoss King
20,704 PointsJust tidied the code up for anyone reading the answers below: