Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial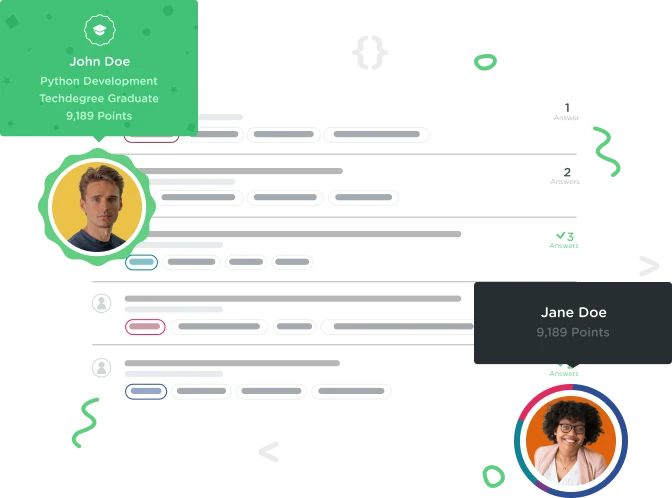
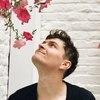
Nikita Voloboev
4,816 PointsHow come this code doesn't get accepted? It gives the correct outputs for me.
I would really be grateful if someone could help me understand what is wrong with it and why it doesn't get accepted to pass the challenge. Perhaps I did something wrong.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
func getPosition() -> Point {
return Point(x: location.x, y: location.y)
}
}
// Enter your code below
class Robot: Machine {
var robotLocation: Point
override init() {
self.robotLocation = Point(x: 0, y: 0)
}
func sayXPos() {
print("\(robotLocation.x)")
}
func increaseXbyOne() {
robotLocation.x = robotLocation.x + 1
}
override func getPosition() -> Point {
return Point(x: robotLocation.x, y: robotLocation.y)
}
override func move(direction: String) {
switch direction {
case "Up":
robotLocation.y = robotLocation.y + 1
case "Down":
robotLocation.y = robotLocation.y - 1
case "Left":
robotLocation.x = robotLocation.x - 1
case "Right":
robotLocation.x = robotLocation.x + 1
default:
break
}
}
}
3 Answers
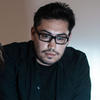
Fernando Boza
25,384 PointsHi Nikita, it should look like this.
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": location.y += 1
case "Down": location.y -= 1
case "Left": location.x -= 1
case "Right": location.x += 1
default: break
}
}
}
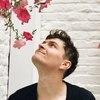
Nikita Voloboev
4,816 PointsThank you, that worked perfectly.
I am really uncertain how super.init works and what exactly does it do and also when do we have to use it.
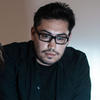
Fernando Boza
25,384 PointsDo you mean override init?
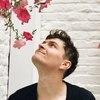
Nikita Voloboev
4,816 PointsYes, for example there was code like this in the tower defence game :
override init(x: Int, y: Int) {
super.init(x: x, y: y)
}
I'm really confused about the super.init and its purpose. From what I understand, when you override something within a subclass, it lets the program know that you want to use that thing instead of what is in its superclasses. Is that correct?
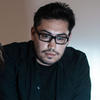
Fernando Boza
25,384 PointsHi Nikita correct. When you override a function within a subclass you also need to override it's initialiser. It can be a bit messy at first but i strongly suggest you watch the video again on inheritance as well read and watch other material online.