Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial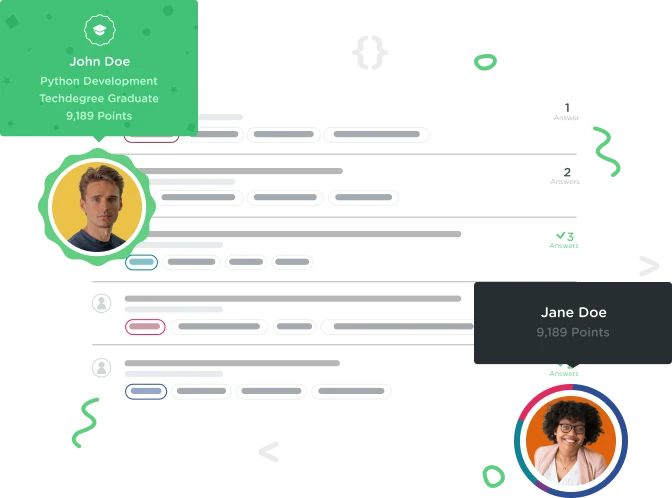
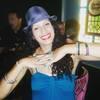
Amber Hoddinott
6,494 PointsHow come this isn't correct?
?
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for (var key in shanghai){
console.log(shanghai.key, ', ', shanghai[values]);
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Objects</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
4 Answers
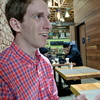
Leyton Parker
6,556 PointsYou need to use the structure of the object like this
for (var key in shanghai) {
console.log(shanghai[key], ', ', shanghai[1]);
}
[1] this being the location of the value [0] this would be the location of the property name

Gunhoo Yoon
5,027 PointsWell there are at least two things that can cause trouble here.
for (var key in shanghai){
console.log(shanghai.key, ', ', shanghai[values]);
}
Object.property or Object[property] can be used to retrieve value of property.
When iterating over object's property use [] to retrieve value instead of dot. (Apparently, I don't have clear explanation for exactly why). But the reason it is better than dot notation is because [] can handle awkward property names better than dot.
You coded shanghai[values] but where is values?
This code probably will come close to your original intention.
for (var key in shanghai){
console.log(key, shanghai[key]);
}

carlin aylsworth
5,650 Pointsvar shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for (var key in shanghai){
console.log(key, ', ', shanghai[key]);
}
"key" has been declared as a standalone variable by the for-in loop so can get it by writing "key". Also, to get the value from "shanghai", you can use the bracket notation "shanghai[key]"

Jacob Mishkin
23,118 PointsYou are really close on this one. here take a look below and let's go over it:
var shanghai = {
population: 14.35e6,
longitude: '31.2000 N',
latitude: '121.5000 E',
country: 'CHN'
};
for (var prop in shanghai) {
console.log(prop, shanghai[prop]);
}
What you need to do is first remove the comma string, challenges are very strict on what they want. next keep with the same variable when iterating through the loop. so instead of [value], use in your case [key]. This will allow the loop to iterate through the var in the for in loop and iterate through the properties.
I hope this helps!