Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial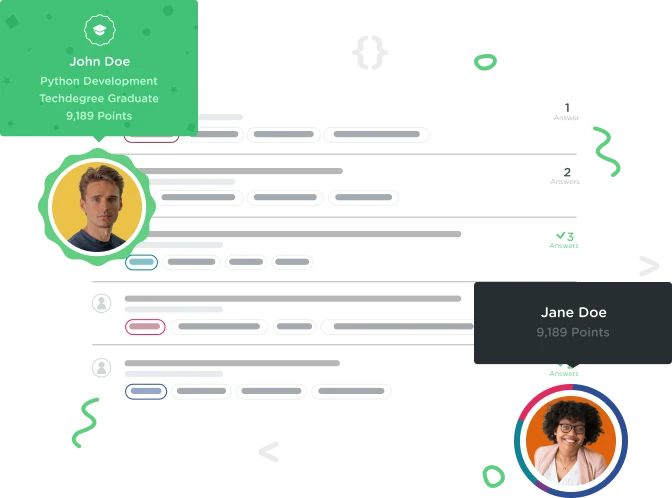

Timothy McCune
6,744 PointsHow come we are using 'AND' instead of '&&'?
I understand that php technically allows the use of AND in conditionals, but it will be deprecated eventually. Using AND to teach the idea of conditionals may make sense, teaching the use of the AND(&&) & OR(||) operator, right off the bat, would avoid any confusion later.
Although there may not seem like there is much of a difference between AND and && -or- OR and || but there are big differences between them. && has a higher precedence over AND. Likewise || has a higher precedence over OR. Take the following example:
// Example #1 $foo = true; $bar = false;
$foobar = $foo and $bar;
You would expect that $foobar would equal false, but surprisingly it would equal true. Why you ask? the = sign has a higher precedence than and therefore php would interpret the above example as: ($foobar = $foo) and $bar;
instead of what we would expect it to do: $foobar = ($foo and $bar);
// Example #2 $foo = true; $bar = false;
$foobar = $foo && $bar; // equals false
The above example works as expected (AND how we hoped the first example would work)
I realize that this lesson is a mere introduction to conditionals in PHP, But wouldn't you agree that it is a good idea to teach good habits from the beginning, rather than introducing bad habits and having them change it later.
Thanks, Tim
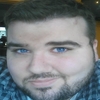
Marcus Parsons
15,719 Points+1 Zachary.
1 Answer
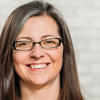
Alena Holligan
Treehouse TeacherThey are both valid. There's the possibility false assignment when using AND as opposed to &&. AND has a lower precedence than &&. So, when having a conditional
<?php $x && $y || $z; ?>
<?php $x AND $y || $z; ?>
In the latter, we're actually executing x and (y or z) since || has a higher precedence then AND.
Now you may say, why not just swap || with OR. Well here's another example of this failing
<?php
$x = true;
$y = false;
$seekingTheTruth = $x and $y;
?>
In this example we can expect the value to return true. This is because the equal operator has higher precedence: ($seekingTheTruth = $x) and $y;
Zachary Green
16,359 PointsZachary Green
16,359 PointsI understand where your coming from. I would guess that the staff just want to be able to assoc AND with && and OR with ||. They do a great job of giving us all the tools but its up to us to use the correct ones in our real world applications.