Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial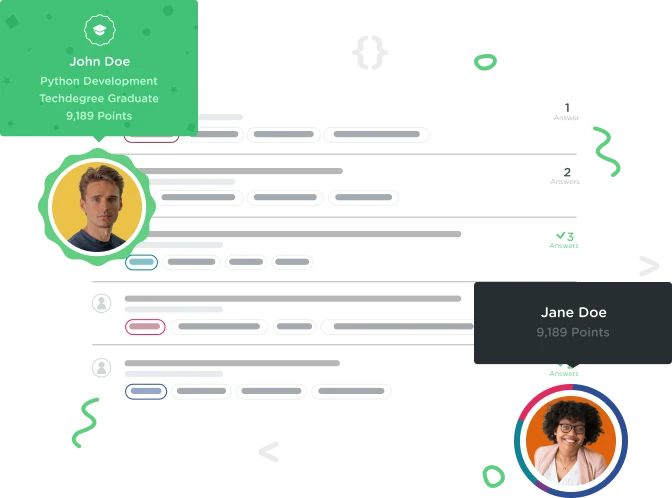
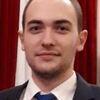
Victor Stanciu
Full Stack JavaScript Techdegree Student 11,196 PointsHow could this be written using the ternary operator?
I tried the code below, but I got an error saying (Bummer: Unexpected token continue):
const percentages = [34, 67, 12, 39, 90, 82, 22, 24, 99];
let upperRange = [];
// upperRange should be: [67, 90, 82, 99]
// Write your code below
percentages.forEach(percentage => (percentage > 50) ? (upperRange.push(percentage)));
2 Answers
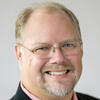
Jason Larson
8,353 PointsYou're getting the error because you haven't given an alternate expression if the statement is false. Personally, I don't think it's beneficial to use a ternary here, as you're not doing anything with the false values, but that's not what you asked. Since you're not doing anything with the values if they are below 50, you can just do null . Try this:
percentages.forEach(percentage => (percentage > 50) ? (upperRange.push(percentage)) : null);
Alternatively, you could do this:
percentages.forEach(percentage => {if (percentage > 50) upperRange.push(percentage)});
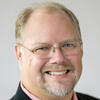
Jason Larson
8,353 PointsHonestly, I'm not 100% sure why continue
doesn't work (and If I'm wrong, hopefully somebody will respond and give us the correct reason), but I suspect it has to do with the fact that continue
is used for breaking out of a loop, and the way the forEach() method is structured, it's not processed in the same way as a standard loop. Since the method calls the function once for each element of the array, there's nowhere for the code to go if it continues. In my mind, I have to think of it more as a series of function calls rather than a loop, and it wouldn't make sense to try to use continue
as a statement by itself in a function.
On the MDN Page for Array.prototype.forEach(), it says "Note: There is no way to stop or break a forEach() loop other than by throwing an exception. If you need such behavior, the forEach() method is the wrong tool."
Victor Stanciu
Full Stack JavaScript Techdegree Student 11,196 PointsVictor Stanciu
Full Stack JavaScript Techdegree Student 11,196 PointsThank you, Jason Larson!
I tried using
continue
for the alternate expression if the statement is false, but looks like I forgot to update the code. I'd really appreciate if you could explain whycontinue
doesn't work also.Anyway, your answer solves my problem!