Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial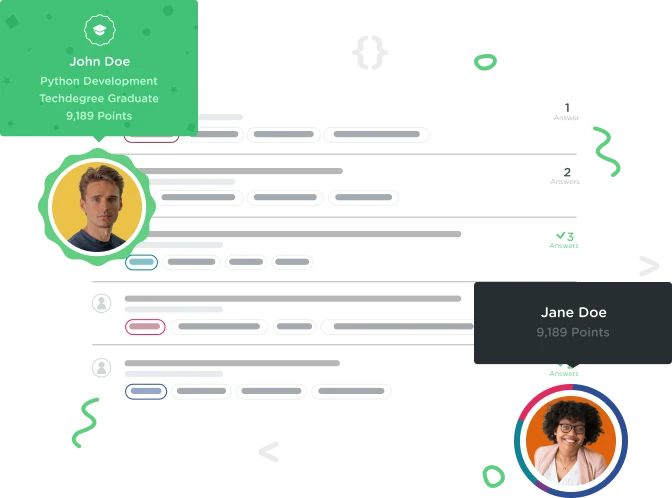
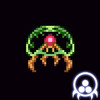
Adam Young
15,791 PointsHow did you complete this "Challenge"?
The challenge for this section of the Python Collections course is as follows:
Write a script that takes for a word (or list of words), removes all of the vowels, and gives the word (or words) back.
For example, if I give the script the word "Treehouse" I should get back "Trhs".
How did you complete this challenge? I love seeing different answers to the same problem.
My code is below, is it 'Pythonic'? Is there any advice you can give to someone learning Python from PHP? Pitfalls to avoid?
def strip_chars(illegal, words):
for word in words:
for char_to_remove in illegal:
word = list(word)
while char_to_remove in word:
index = word.index(char_to_remove)
del word[index]
print(''.join(word))
words = ['car','truck','milkshake','fries','poop', 'ddd', 'vroooomeeeiiiaa']
letters = ['a','e','i','o','u']
strip_chars(letters, words)
3 Answers
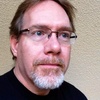
Chris Freeman
Treehouse Moderator 68,423 PointsI like the surgical precision of digging down to the very core of comparing each illegal" char to every char in a word:
def strip_chars(illegal, words):
# for each word...
for word in words:
# walk-through each illegal char
for char_to_remove in illegal:
# create a list of word characters
word = list(word)
# grind through the word, searching and destroying each illegal char
while char_to_remove in word:
index = word.index(char_to_remove)
del word[index]
# put the patient word back together
print(''.join(word))
This is solid coding, but there are short cuts. The power of the keyword in
is like a laparoscopic surgury. It allows you to inspect an object with out major sugury.
Instead of breaking it all down to compare char by char, you can simply ask if a letter is "In" a container:
def strip_chars(illegal, words):
# for each word...
for word in words:
# walk-through each char in word
new_word = []
for char in word:
# check if illegal
if char not in illegal:
new_word.append(char)
# put the patient word back together
print(''.join(new_word))
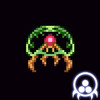
Adam Young
15,791 PointsExactly the kind of feedback/solution I was looking for! I have to keep in mind that 'is', 'not', and 'in' are in my toolbox now. Thanks for the kind words and the very insightful advice!

Lofton Newton
222 PointsCan make this more concise by using list comprehensions.
VOWELS = ['a','e','i','o','u']
def strip_vowels_from_word(word, vowels):
return ''.join([ letter for letter in word if letter.lower() not in vowels ])
def strip_vowels_from_words(words, vowels=VOWELS):
return [ strip_vowels_from_word(word, vowels) for word in words ]
words = ['car','truck','milkshake','fries','poop', 'ddd', 'vroooomeeeiiiaa']
strip_vowels_from_words(words)

Benedictt Vasquez
2,130 PointsHere a other way:
def borrar_vocales(y):
a = list(y)
x = len(a)
while True:
for letter in a:
if letter in ('a', 'e', 'i', 'o', 'u'):
a.remove(letter)
x = x-1
if x < 0:
break
z = ''.join(a)
print(z)
Adam Young
15,791 PointsAdam Young
15,791 PointsApparently the guy that knows Python knows it better than me. The pop() method would sure work wonders here...