Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial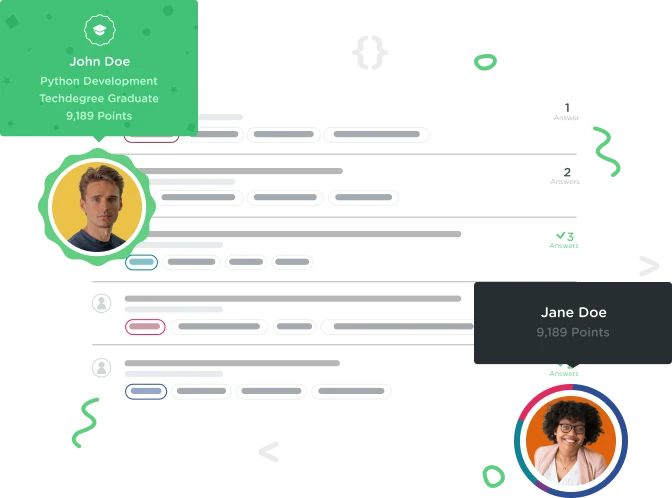

ian sobo
3,851 PointsHow did you do Array Madness Extra Credit (found in 'coding the fun facts' section)?
Hey guys, i was just wondering how you guys went about accomplishing the Array Madness Extra Credit task? Here's how I did it. If you have any constructive criticism, comments, or would like to point out if something is inefficient, weird, or not a good practice, PLEASE LET ME KNOW! Share your methods below and your reasoning!
package com.example.ian.myapplication;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.TextView;
import java.lang.reflect.Array;
import java.util.Arrays;
public class MainActivity extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TextView intArrayFwd = (TextView) findViewById(R.id.intArrayFwd);
TextView intArrayBkwd = (TextView) findViewById(R.id.intArrayBkwd);
int[] intArray = {0,1,2,3,4,5,6,7,8,9};
String stringArray = Arrays.toString(intArray);
intArrayFwd.setText(stringArray);
StringBuilder stringBuilder = new StringBuilder(stringArray).reverse();
intArrayBkwd.setText(stringBuilder);
}
}
This works, almost perfectly, except for the fact that even the brackets are reversed haha. I could've gone about using a loop, but i wanted to see if there were any useful built-in methods that would assist me, and thanks to stackoverflow, i found the very useful class of StringBuild and StringBuffer.
1 Answer

ABRAHAM CHAVEZ REYES
361 Pointspackage com.example.hp.testlol;
import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.ImageButton; import android.widget.TextView;
import java.util.Arrays;
public class Mtest extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_mtest);
final TextView firs=(TextView)findViewById(R.id.TV);
final TextView second=(TextView)findViewById(R.id.TV2);
ImageButton img1= (ImageButton)findViewById(R.id.img1);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
int[] numbersArray = { 5,7,3,0,1,2,8,9,4,6 };
Arrays.sort(numbersArray);
for (int a = 0; a < numbersArray.length; a++) {
firs.append(String.valueOf(numbersArray[a]));
}
for (int b = numbersArray.length - 1; b > -1; b--) {
second.append(String.valueOf(numbersArray[b]));
}
}
};
img1.setOnClickListener(listener);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.mtest, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
this is the way I did it. but when i press the button i get the whole array of numbers at the same time. i wold like to know if someone make just one number at a time not the whole array of numbers at the same click of button.
Nick Frankel
428 PointsNick Frankel
428 PointsI spent ages trying to think about how to do this without using a loop as the lectures so far don't really cover this. Not much else to say as i'm a newbie too but good work for researching it!