Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial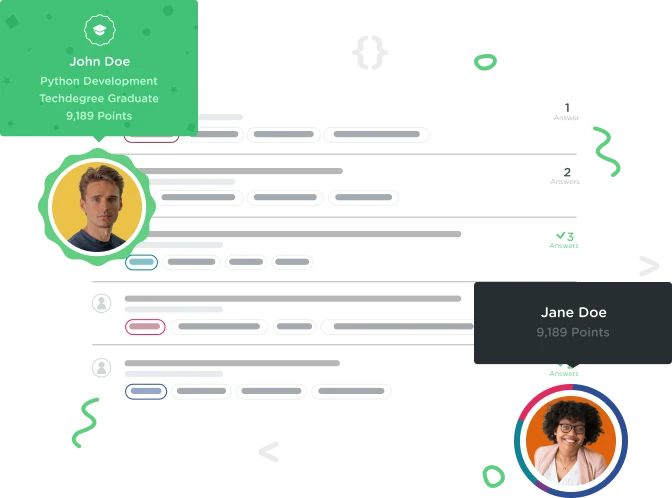

Matthew Dabell
4,558 PointsHow do I add a map marker to the user's location (android)?
I have followed the tutorial here:
http://blog.teamtreehouse.com/beginners-guide-location-android
However, the location is only marked on the map if the code is typed into the setUpMap() method like so:
private void setUpMap() { map.addMarker(new MarkerOptions().position(new LatLng(0, 0)).title("Marker")); }
And then it obviously only comes up on 0,0. However, the marker should be added by handleNewLocation(Location location).
Has anyone does this tutorial and gotten it to work? Or does anyone know how to add a marker to the map that brings up the user's location?
Rami Awar
5,435 PointsRami Awar
5,435 PointsYeah i got it to work. Here's the whole code I added some things on my own like checking if location services are available and what not, but you can just take what you need.