Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial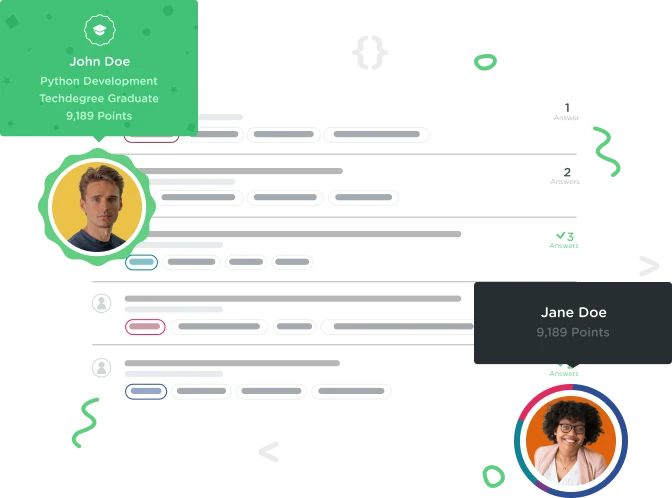

Adrian Yao
1,002 PointsHow do I append?
i think i get the switch part but not how to append it to the array. Thanks for the help.
var europeanCapitals: [String] = []
var asianCapitals: [String] = []
var otherCapitals: [String] = []
let world = [
"BEL": "Brussels",
"LIE": "Vaduz",
"BGR": "Sofia",
"USA": "Washington D.C.",
"MEX": "Mexico City",
"BRA": "Brasilia",
"IND": "New Delhi",
"VNM": "Hanoi"]
for (key, value) in world {
// Enter your code below
switch world {
case "BEL": print("Brussels") .append europeanCapitals
case "LIE": print("LIE") .append euopeanCapitals
case "BGR": print("BGR") .append euopeanCapitals
case "IND": print("New Delhi").append asianCapitals
case "VNM": print("Hanoi") .append asianCapitals
default: .append otherCapitals
// End code
}
1 Answer
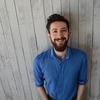
Matt Skelton
4,548 PointsHey Adrian,
Good effort so far, I'm more than happy to run you through how exactly the append functionality works but first, let's take a closer look at your switch statement.
You're very nearly there, the first problem that stops us progressing is that we're switching on the wrong value. The task requires us to do the following:
To do this you're going to use a switch statement and switch on the key. For cases where the key is a European country, append the value (not the key!) to the europeanCapitals array.
At the moment however, we're switching on the world value, which is of type [String: String]. We want to switch on the key, and use these keys to decide which arrays we want to assign our values to.
Next, let's take a look at how we can append values to our arrays.
for (key, value) in world
{
switch key
{
case "BEL": europeanCapitals.append(value)
case "IND": asianCapitals.append(value)
default: otherCapitals.append(value)
// More Cases Here
}
}
Here's an example of how the append functionality works. We call the append function on the array we wish to add our capital city to. As the task wants us to append the name (or value) of a city to our array, we pass in the value of our key value pair.
Hopefully this points you in the right direction as to how to use the append functionality. Give it a try filling out the other cases to accommodate each of the capital cities.
A few final tips, double check your curly braces match up, you're currently missing a closing curly brace. And ensure the spelling of your variables are correct. The compiler can be very particular when it comes to spelling (in particular, your europeanCaptials variable).
Finally, if you're feeling particularly adventurous, you can have a go at combining your switch statements to condense your code a little bit more. This isn't compulsory so don't feel forced into it, but it can make your code a little more readable:
for (key, value) in world
{
switch key
{
case "BEL", "LIE", "BGR": europeanCapitals.append(value)
// More Cases Here
}
}
Good luck, feel free to let me know if you have any more problems!
Melanie Gershman
4,425 PointsMelanie Gershman
4,425 PointsA couple of things are off here: 1) you're not being asked to print the single city, you're expected to return an array of strings of cities that correspond to an array. to address this issue, think about what you want to happen in each case in terms of control flow. you are using the keys of your 'world' dictionary as your case. so when we get our first key, "BEL", you want it to be added to your europeanCapitals array. meaning, in that case, we're going to append the value ("Brussels") to the europeanCapitals array. We use switch statements to do a different thing based on the element we are evaluating in our loop. check out the below:
2) another thing to consider is what you've written above. you're calling the print method and then trying to run another method on top of that, which is probably causing some errors. it seems like append is a method to be used on an array type. so we call append on the array, and pass in the item we want to append as an argument. it looks like you've gone about it the other way around.
3) syntactically, you want to make sure you don't have a space between append and the parentheses' through which you're passing in the argument.
i hope that was clear, take a look at the docs to get a refresher on how switch statements should look, and at the code sample above.