Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial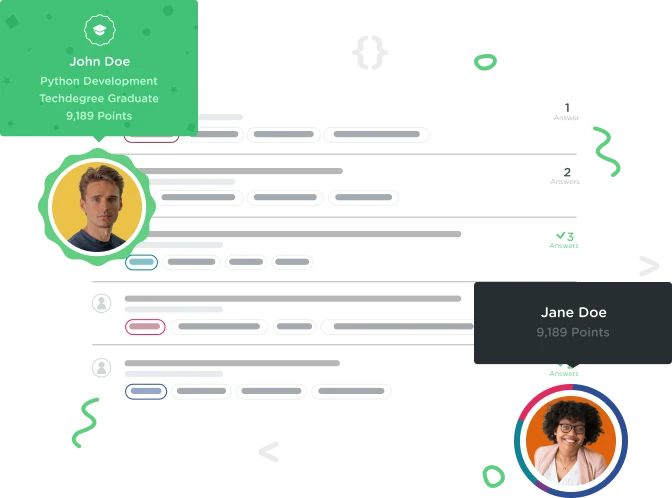

Adrian Yao
1,002 PointsHow do I append an array here?
I dont understand what this problem is asking. Clarification would be great. Thanks!
// Enter your code below
var results: [Int] = [.append ()]
for multiplier in 1...10 {
print(" \(multiplier) times 6 is equal to \(multiplier * 6)")
}
2 Answers
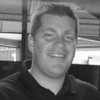
Mike Hickman
19,817 PointsHey Adrian,
So, the basic idea is now that you've created the empty for loop, you're going to append a number to the results array each time the loop runs (as it goes 1 - 10).
Your for loop is correct, but you messed up your results array as you've been working through the problem.
Let's start fresh at the beginning of the step 2 challenge:
var results: [Int] = []
K. We have an empty results array. Perfect.
for multiplier in 1...10 {
}
Cool. We're back to the empty for loop that you created in step 1. Now, literally the only thing it wants you to do is append a number with each run of the for loop into the results array. Think of it as literally just adding a number into a list in the array. Example: results = [1, 2, 3, 4]. Luckily, we can use the .append
method to do this.
Just in case you're newer to loops, the way ours is setup is saying "every time we run through the loop, whatever number in the range (1-10) we're on, that number is called "multiplier"
for multiplier in 1...10 {
// grab the results array and append something
results.append()
}
We want to append 6 * whatever the current number ("multiplier") is as we do the loop.
for multiplier in 1...10 {
// let's throw the actual multiplication in now to finish it off
results.append(multiplier * 6)
}
So, when we start the loop, it'll be results.append(1 * 6)
, then results.append(2 * 6)
in the next loop. Each time the loop runs we're adding a number to the list in the results array.
Hopefully I didn't OVER explain it..just wanted to help you understand in case you're new to all of this.
Have fun! Mike

O'Ryan Alexander
Full Stack JavaScript Techdegree Student 5,671 Pointsyou got to use the .append method:
results.append(multiplier * 6)
Adrian Yao
1,002 PointsAdrian Yao
1,002 Pointsvar results: [Int] = [.append (multiplier)*6)]
I am still confused sorry, it still says it cant be compiled. Thanks for the help!
Mike Hickman
19,817 PointsMike Hickman
19,817 PointsAdrian Yao - It's fine. Here's a follow-up to my first answer after seeing your comment.
I think you're still caught up on the first line. Put the first line exactly like I have it and don't touch it (don't even think about it again). All that line is doing is setting up an array to a variable called
results
. You do the actual appending/adding to the array from within the for loop (my last code block in my first answer is literally all you need to run the for loop correctly). Below is the entire thing together.Read through my explanation again before you move on to make sure you understand before the code gets harder down the line :)
You'll hit a million things you don't understand as you move along and that's just part of the coding journey. Just keep pushing through and ask people questions.
Cheers, Mike